Deck 2: Instructions
سؤال
سؤال
سؤال
سؤال
سؤال
سؤال
سؤال
سؤال
سؤال
سؤال
سؤال
سؤال
فتح الحزمة
قم بالتسجيل لفتح البطاقات في هذه المجموعة!
Unlock Deck
Unlock Deck
1/12
العب
ملء الشاشة (f)
Deck 2: Instructions
1
In the snippet of MIPS assembler code below, how many times is instruction memory accessed? How many times is data memory accessed? (Count only accesses to memory, not registers.) 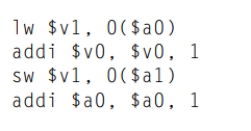
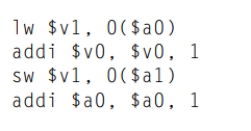
The instruction memory is accessed four times (as there are four instructions) and the data memory is
accessed twice (once for the lw instruction and another time for the sw instruction).
accessed twice (once for the lw instruction and another time for the sw instruction).
2
Some machines have a special flag register which contains status bits. These bits often include the carry and overflow bits. Describe the difference between the functionality of these two bits and give an example of an arithmetic operation that would lead to them being set to different values.
The carry flag is set when arithmetic operation results in generating a carry bit out of the most significant bit position of its operand. The overflow flag is set when an arithmetic operation results in generating a carry bit out of the most significant bit position of the physical register which holds its operand. An overflow means that the register size is not big enough to hold the result of the current arithmetic operation while a carry just indicates that the resulting value's most significant bit position is higher (or lower in case of a borrow) than its operand by a bit position. When we add two integers: 0x0100 and 0x0110 which are held in 16-bit registers, the result 0x1010 generates a carry but not a overflow bit.
3
Convert the C function below to MIPS assembly language. Make sure that your assembly language code could be called from a standard C program (that is to say, make sure you follow the MIPS calling conventions).
unsigned int sum(unsigned int n)
{
if (n == 0) return 0; else return n + sum(n-1);
}
This machine has no delay slots. The stack grows downward (toward lower memory addresses). The following registers are used in the calling convention: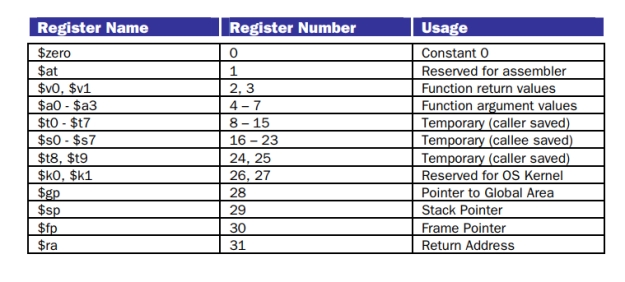
unsigned int sum(unsigned int n)
{
if (n == 0) return 0; else return n + sum(n-1);
}
This machine has no delay slots. The stack grows downward (toward lower memory addresses). The following registers are used in the calling convention:
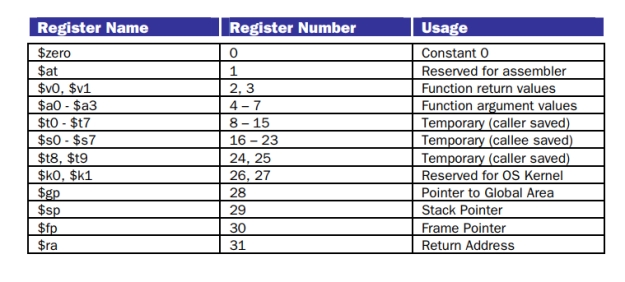
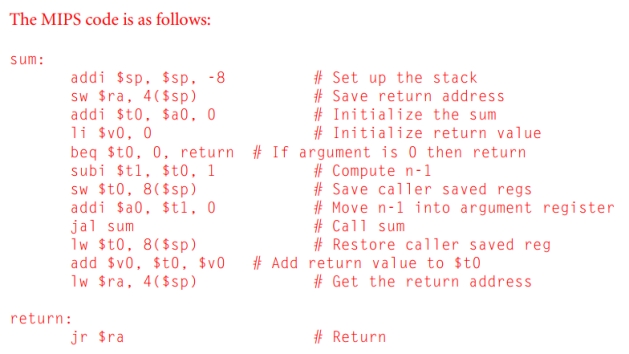
4
The MIPS instruction set includes several shift instructions. They include logical-shift- left, logical-shift-right, and arithmetic-shift-right. Other architectures only provide an arithmetic- shift-right instruction.
a) Why doesn't MIPS offer an "arithmetic-shift-left" opcode?
b)How would you implement in the assembler a logical-shift-left (LSL) pseudo-operation for a machine that didn't have this particular instruction? Be sure your LSL instruction can shift up to W- bits where W is the machine word size in bits.
a) Why doesn't MIPS offer an "arithmetic-shift-left" opcode?
b)How would you implement in the assembler a logical-shift-left (LSL) pseudo-operation for a machine that didn't have this particular instruction? Be sure your LSL instruction can shift up to W- bits where W is the machine word size in bits.
فتح الحزمة
افتح القفل للوصول البطاقات البالغ عددها 12 في هذه المجموعة.
فتح الحزمة
k this deck
5
In MIPS assembly, write an assembly language version of the following C code segment:
for (i = 0; i < 98; i ++) {
C[i] = A[i + 1] - A[i] * B[i + 2]
}
Arrays A, B and C start at memory location A000hex, B000hex and C000hex respectively. Try to reduce the total number of instructions and the number of expensive instructions such as multiplies.
for (i = 0; i < 98; i ++) {
C[i] = A[i + 1] - A[i] * B[i + 2]
}
Arrays A, B and C start at memory location A000hex, B000hex and C000hex respectively. Try to reduce the total number of instructions and the number of expensive instructions such as multiplies.
فتح الحزمة
افتح القفل للوصول البطاقات البالغ عددها 12 في هذه المجموعة.
فتح الحزمة
k this deck
6
Use the register and memory values in the table below for the next questions. Assume a 32-bit machine. Assume each of the following questions starts from the table values; that is, DO NOT use value changes from one question as propagating into future parts of the question.
a) Give the values of R1, R2, and R3 after this instruction: add R3, R2, R1
b) What values will be in R1 and R3 after this instruction is executed: load R3, 12(R1)
c) What values will be in the registers after this instruction is executed: addi R2, R3, #16
a) Give the values of R1, R2, and R3 after this instruction: add R3, R2, R1
b) What values will be in R1 and R3 after this instruction is executed: load R3, 12(R1)
c) What values will be in the registers after this instruction is executed: addi R2, R3, #16
فتح الحزمة
افتح القفل للوصول البطاقات البالغ عددها 12 في هذه المجموعة.
فتح الحزمة
k this deck
7
Loop Unrolling and Fibonacci: Consider the following pseudo-C code to compute the fifth Fibonacci number (F(5)).
1 int a,b,i,t;
2 a=b=1; /* Set a and b to F(2) and F(1) respectively */ 3 for(i=0;i<2;i++)
4 {
5 t=a; /* save F(n-1) to a temporary location */ 6 a+=b; /* F(n) = F(n-1) + F(n-2) */
7 b=t; /* set b to F(n-1) */ 8 }
One observation that a compiler might make is that the loop construction is somewhat unnecessary. Since the the range of the loop indices is fixed, one can unroll the loop by simply writing three iterations of the loop one after the other without the intervening increment/comparison on i. For example, the above could be written as:
1 int a,b,t;
2 a=b=1;
3 t=a;
4 a+=b;
5 b=t;
6 t=a;
7 a+=b;
8 b=t;
(a) Convert the pseudo-C code for both of the snippets above into reasonably efficient MIPS code. Represent each variable of the pseudo-C program with a register. Try to follow the pseudo-C code as closely as possible (i.e. the first snippet should have a loop in it, while the second should not).
(b) Now suppose that instead of the fifth Fibonacci number we decided to compute the 20th. How many static instructions would there be in the first version and how many would there be in the unrolled version? What about dynamic instructions? You do not need to write out the assembly for this part.
1 int a,b,i,t;
2 a=b=1; /* Set a and b to F(2) and F(1) respectively */ 3 for(i=0;i<2;i++)
4 {
5 t=a; /* save F(n-1) to a temporary location */ 6 a+=b; /* F(n) = F(n-1) + F(n-2) */
7 b=t; /* set b to F(n-1) */ 8 }
One observation that a compiler might make is that the loop construction is somewhat unnecessary. Since the the range of the loop indices is fixed, one can unroll the loop by simply writing three iterations of the loop one after the other without the intervening increment/comparison on i. For example, the above could be written as:
1 int a,b,t;
2 a=b=1;
3 t=a;
4 a+=b;
5 b=t;
6 t=a;
7 a+=b;
8 b=t;
(a) Convert the pseudo-C code for both of the snippets above into reasonably efficient MIPS code. Represent each variable of the pseudo-C program with a register. Try to follow the pseudo-C code as closely as possible (i.e. the first snippet should have a loop in it, while the second should not).
(b) Now suppose that instead of the fifth Fibonacci number we decided to compute the 20th. How many static instructions would there be in the first version and how many would there be in the unrolled version? What about dynamic instructions? You do not need to write out the assembly for this part.
فتح الحزمة
افتح القفل للوصول البطاقات البالغ عددها 12 في هذه المجموعة.
فتح الحزمة
k this deck
8
Prior to the early 1980s, machines were built with more and more complex instruction set. The MIPS is a RISC machine. Why has there been a move to RISC machines away from complex instruction machines?
فتح الحزمة
افتح القفل للوصول البطاقات البالغ عددها 12 في هذه المجموعة.
فتح الحزمة
k this deck
9
Consider the following assembly code for parts 1 and 2.
r1 = 99
Loop:
r1 = r1 - 1
branch r1 > 0, Loop halt
(a) During the execution of the above code, how many dynamic instructions are executed?)
(b) Assuming a standard unicycle machine running at 100 KHz, how long will the above code take to complete?
r1 = 99
Loop:
r1 = r1 - 1
branch r1 > 0, Loop halt
(a) During the execution of the above code, how many dynamic instructions are executed?)
(b) Assuming a standard unicycle machine running at 100 KHz, how long will the above code take to complete?
فتح الحزمة
افتح القفل للوصول البطاقات البالغ عددها 12 في هذه المجموعة.
فتح الحزمة
k this deck
10
Suppose that a new MIPS instruction, called bcp, was designed to copy a block of words from one address to another. Assume that this instruction requires that the starting address of the source block be in register $t1 and that the destination address be in $t2. The instruction also requires that the number of words to copy be in $t3 (which is > 0). Furthermore, assume that the values of these registers as well as register $t4 can be destroyed in executing this instruction (so that the registers can be used as temporaries to execute the instruction).
Do the following: Write the MIPS assembly code to implement a block copy without this instruction. Write the MIPS assembly code to implement a block copy with this instruction. Estimate the total cycles necessary for each realization to copy 100-words on the multicycle machine.
Do the following: Write the MIPS assembly code to implement a block copy without this instruction. Write the MIPS assembly code to implement a block copy with this instruction. Estimate the total cycles necessary for each realization to copy 100-words on the multicycle machine.
فتح الحزمة
افتح القفل للوصول البطاقات البالغ عددها 12 في هذه المجموعة.
فتح الحزمة
k this deck
11
Write the following sequence of code into MIPS assembler:
x = x + y + z - q;
Assume that x, y, z, q are stored in registers $s1-$s4.
x = x + y + z - q;
Assume that x, y, z, q are stored in registers $s1-$s4.
فتح الحزمة
افتح القفل للوصول البطاقات البالغ عددها 12 في هذه المجموعة.
فتح الحزمة
k this deck
12
In MIPS assembly, write an assembly language version of the following C code segment:
int A[100], B[100];
for (i=1; i < 100; i++) { A[i] = A[i-1] + B[i];
}
At the beginning of this code segment, the only values in registers are the base address of arrays A and B
in registers $a0 and $a1. Avoid the use of multiplication instructions-they are unnecessary.
int A[100], B[100];
for (i=1; i < 100; i++) { A[i] = A[i-1] + B[i];
}
At the beginning of this code segment, the only values in registers are the base address of arrays A and B
in registers $a0 and $a1. Avoid the use of multiplication instructions-they are unnecessary.
فتح الحزمة
افتح القفل للوصول البطاقات البالغ عددها 12 في هذه المجموعة.
فتح الحزمة
k this deck