Deck 10: Arraylists
Question
Question
Question
Question
Question
Question
Question
Unlock Deck
Sign up to unlock the cards in this deck!
Unlock Deck
Unlock Deck
1/7
Play
Full screen (f)
Deck 10: Arraylists
1
Programming
Write a static method named longestName that reads names typed by the user and prints the longest name (the name that contains the most characters) in the format shown below. Your method should accept a console Scanner and an integer n as parameters and should then prompt for n names.
The longest name should be printed with its first letter capitalized and all subsequent letters in lowercase, regardless of the capitalization the user used when typing in the name.
If there is a tie for longest between two or more names, use the tied name that was typed earliest. Also print a message saying that there was a tie, as in the right log below. It's possible that some shorter names will tie in length, such as DANE and Erik in the left log below; but don't print a message unless the tie is between the longest names.
You may assume that n is at least 1, that each name is at least 1 character long, and that the user will type single-word names consisting of only letters. The following table shows two sample calls and their output.
(extra writing space for Problem #6)
Write a static method named longestName that reads names typed by the user and prints the longest name (the name that contains the most characters) in the format shown below. Your method should accept a console Scanner and an integer n as parameters and should then prompt for n names.
The longest name should be printed with its first letter capitalized and all subsequent letters in lowercase, regardless of the capitalization the user used when typing in the name.
If there is a tie for longest between two or more names, use the tied name that was typed earliest. Also print a message saying that there was a tie, as in the right log below. It's possible that some shorter names will tie in length, such as DANE and Erik in the left log below; but don't print a message unless the tie is between the longest names.
You may assume that n is at least 1, that each name is at least 1 character long, and that the user will type single-word names consisting of only letters. The following table shows two sample calls and their output.
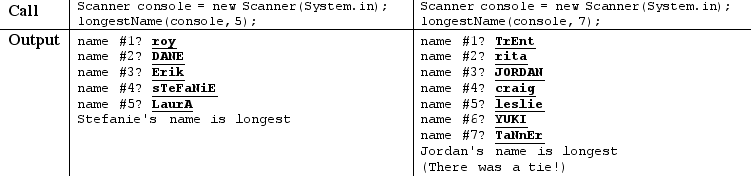
There are many ways to solve any programming problem. Here are three common correct solutions we saw:
// longer solution; pull first post out of fencepost
public static void longestName(Scanner console, int names) {
System.out.print("name #1? ");
String longest = console.next();
int count = 1;
for (int i = 2; i <= names; i++) {
System.out.print("name #" + i + "? ");
String name = console.next();
if (name.length() == longest.length()) {
count++;
}
if (name.length() > longest.length()) {
longest = name;
count = 1;
}
}
String fixedName = longest.substring(0, 1).toUpperCase();
fixedName = fixedName + longest.substring(1).toLowerCase();
System.out.println(fixedName + "'s name is longest");
if (count > 1) {
System.out.println("(There was a tie!)");
}
}
// shorter solution; boolean flag
public static void longestName(Scanner console, int names) {
String longest = "";
boolean tie = false;
for (int i = 1; i <= names; i++) {
System.out.print("name #" + i + "? ");
String name = console.next();
if (name.length() > longest.length()) {
longest = name;
tie = false;
} else if (name.length() == longest.length()) {
tie = true;
}
}
longest = longest.substring(0, 1).toUpperCase() + longest.substring(1).toLowerCase();
System.out.println(longest + "'s name is longest");
if (tie) {
System.out.println("(There was a tie!)");
}
}
// store longest two strings and compare
public static void longestName(Scanner console, int n) {
String longest = "";
String longest2 = "";
for (int i = 1; i <= n; i++) {
System.out.print("name #" + i + "? ");
String name = console.next();
if (name.length() > longest.length()) {
longest = name;
longest2 = name;
} else if (name.length() == longest.length()) {
longest2 = name;
}
}
String capitalized = longest.toUpperCase().charAt(0) + longest.toLowerCase().substring(1);
System.out.println(capitalized + "'s name is longest");
if (!longest.equalsIgnoreCase(longest2)) {
System.out.println("(There was a tie!)");
}
}
// longer solution; pull first post out of fencepost
public static void longestName(Scanner console, int names) {
System.out.print("name #1? ");
String longest = console.next();
int count = 1;
for (int i = 2; i <= names; i++) {
System.out.print("name #" + i + "? ");
String name = console.next();
if (name.length() == longest.length()) {
count++;
}
if (name.length() > longest.length()) {
longest = name;
count = 1;
}
}
String fixedName = longest.substring(0, 1).toUpperCase();
fixedName = fixedName + longest.substring(1).toLowerCase();
System.out.println(fixedName + "'s name is longest");
if (count > 1) {
System.out.println("(There was a tie!)");
}
}
// shorter solution; boolean flag
public static void longestName(Scanner console, int names) {
String longest = "";
boolean tie = false;
for (int i = 1; i <= names; i++) {
System.out.print("name #" + i + "? ");
String name = console.next();
if (name.length() > longest.length()) {
longest = name;
tie = false;
} else if (name.length() == longest.length()) {
tie = true;
}
}
longest = longest.substring(0, 1).toUpperCase() + longest.substring(1).toLowerCase();
System.out.println(longest + "'s name is longest");
if (tie) {
System.out.println("(There was a tie!)");
}
}
// store longest two strings and compare
public static void longestName(Scanner console, int n) {
String longest = "";
String longest2 = "";
for (int i = 1; i <= n; i++) {
System.out.print("name #" + i + "? ");
String name = console.next();
if (name.length() > longest.length()) {
longest = name;
longest2 = name;
} else if (name.length() == longest.length()) {
longest2 = name;
}
}
String capitalized = longest.toUpperCase().charAt(0) + longest.toLowerCase().substring(1);
System.out.println(capitalized + "'s name is longest");
if (!longest.equalsIgnoreCase(longest2)) {
System.out.println("(There was a tie!)");
}
}
2
Parameter Mystery
At the bottom of the page, write the output produced by the following program, as it would appear on the console.
public class ParameterMystery {
public static void main(String[] args) {
String literal = "8";
String brace = "semi";
String paren = brace;
String semi = "brace";
String java = "42";
param(java, brace, semi);
param(literal, paren, java);
param(brace, semi, "literal");
param("cse", literal + 4, "1");
}
public static void param(String semi, String java, String brace) {
System.out.println(java + " missing a " + brace + " and " + semi);
}
}
At the bottom of the page, write the output produced by the following program, as it would appear on the console.
public class ParameterMystery {
public static void main(String[] args) {
String literal = "8";
String brace = "semi";
String paren = brace;
String semi = "brace";
String java = "42";
param(java, brace, semi);
param(literal, paren, java);
param(brace, semi, "literal");
param("cse", literal + 4, "1");
}
public static void param(String semi, String java, String brace) {
System.out.println(java + " missing a " + brace + " and " + semi);
}
}
semi missing a brace and 42
semi missing a 42 and 8
brace missing a literal and semi
84 missing a 1 and cse
semi missing a 42 and 8
brace missing a literal and semi
84 missing a 1 and cse
3
While Loop Simulation
For each call below to the following method, write the output that is produced, as it would appear on the console:
public static void whileMystery(int x, int y) {
int z = 0;
while (x % y != 0) {
x = x / y;
z++;
System.out.print(x + ", ");
}
System.out.println(z);
}
For each call below to the following method, write the output that is produced, as it would appear on the console:
public static void whileMystery(int x, int y) {
int z = 0;
while (x % y != 0) {
x = x / y;
z++;
System.out.print(x + ", ");
}
System.out.println(z);
}
4
Programming
Write a static method named largerDigits that accepts two integer parameters a and b and returns a new integer c where each digit of c gets its value from the larger of a's and b's digit in the same place. That is, the ones digit of c is the larger of the ones digit of a and the ones digit of b, and the tens digit of c is the larger of the tens digit of a and the tens digit of b, and so on. You may assume that a and b are positive integers (greater than 0).
For example, suppose a is 603452384 and b is 921782. Their digits would be combined as follows to produce c:
a 603452380
b 920784
------------------
c 952784 (return value)
Notice that if a particular digit place is absent from one number or the other, such as the 603 at the start of a above, no digit is carried over to c. The following table lists some more calls to your method and their expected return values:
Hint: If you are building a result number, you may need to use Math.pow or accumulate a multiplier with each digit.
You may not use a String to solve this problem.
Write a static method named largerDigits that accepts two integer parameters a and b and returns a new integer c where each digit of c gets its value from the larger of a's and b's digit in the same place. That is, the ones digit of c is the larger of the ones digit of a and the ones digit of b, and the tens digit of c is the larger of the tens digit of a and the tens digit of b, and so on. You may assume that a and b are positive integers (greater than 0).
For example, suppose a is 603452384 and b is 921782. Their digits would be combined as follows to produce c:
a 603452380
b 920784
------------------
c 952784 (return value)
Notice that if a particular digit place is absent from one number or the other, such as the 603 at the start of a above, no digit is carried over to c. The following table lists some more calls to your method and their expected return values:
Hint: If you are building a result number, you may need to use Math.pow or accumulate a multiplier with each digit.
You may not use a String to solve this problem.
Unlock Deck
Unlock for access to all 7 flashcards in this deck.
Unlock Deck
k this deck
5
Assertions
For each of the five points labeled by comments, identify each of the assertions in the table below as either being always true, never true, or sometimes true / sometimes false. (You may abbreviate them as A, N, or S.)
public static int count(int n) {
int even = 0;
int odd = 0;
// Point A
while (n != 0 && even <= odd) {
if (n % 2 == 0) {
even++;
// Point B
} else {
// Point C
odd++;
}
n = n / 2;
// Point D
}
// Point E
return even - odd;
}
For each of the five points labeled by comments, identify each of the assertions in the table below as either being always true, never true, or sometimes true / sometimes false. (You may abbreviate them as A, N, or S.)
public static int count(int n) {
int even = 0;
int odd = 0;
// Point A
while (n != 0 && even <= odd) {
if (n % 2 == 0) {
even++;
// Point B
} else {
// Point C
odd++;
}
n = n / 2;
// Point D
}
// Point E
return even - odd;
}
Unlock Deck
Unlock for access to all 7 flashcards in this deck.
Unlock Deck
k this deck
6
If/Else Simulation
For each call below to the following method, write the output that is produced, as it would appear on the console:
public static void ifElseMystery(int a, int b, int c) {
if (a < b && a < c) {
a = a + c;
c++;
} else if (a >= b) {
a = a - b;
b--;
}
if (a >= b && a >= c) {
a++;
c++;
}
System.out.println(a + " " + b + " " + c);
}
For each call below to the following method, write the output that is produced, as it would appear on the console:
public static void ifElseMystery(int a, int b, int c) {
if (a < b && a < c) {
a = a + c;
c++;
} else if (a >= b) {
a = a - b;
b--;
}
if (a >= b && a >= c) {
a++;
c++;
}
System.out.println(a + " " + b + " " + c);
}
Unlock Deck
Unlock for access to all 7 flashcards in this deck.
Unlock Deck
k this deck
7
Expressions
For each expression at left, indicate its value in the right column. List a value of appropriate type and capitalization.
e.g., 7 for an int, 7.0 for a double, "hello" for a String, true or false for a boolean.
2 \& \& 1>3) & \underline{ \text { }} \underline{ \text { }} \underline{ \text { }} \underline{ \text { }} \underline{ \text { }} \underline{ \text { }}\\1 / 2+-(157 / 10 / 10.0)+9.0 * 1 / 2 & \underline{ \text { }} \underline{ \text { }} \underline{ \text { }} \underline{ \text { }} \underline{ \text { }} \underline{ \text { }} \\24 \div 5+9 \div(6 \div 4) & \underline{ \text { }} \underline{ \text { }} \underline{ \text { }} \underline{ \text { }} \underline{ \text { }} \underline{ \text { }} \end{array}">
For each expression at left, indicate its value in the right column. List a value of appropriate type and capitalization.
e.g., 7 for an int, 7.0 for a double, "hello" for a String, true or false for a boolean.
2 \& \& 1>3) & \underline{ \text { }} \underline{ \text { }} \underline{ \text { }} \underline{ \text { }} \underline{ \text { }} \underline{ \text { }}\\1 / 2+-(157 / 10 / 10.0)+9.0 * 1 / 2 & \underline{ \text { }} \underline{ \text { }} \underline{ \text { }} \underline{ \text { }} \underline{ \text { }} \underline{ \text { }} \\24 \div 5+9 \div(6 \div 4) & \underline{ \text { }} \underline{ \text { }} \underline{ \text { }} \underline{ \text { }} \underline{ \text { }} \underline{ \text { }} \end{array}">
Unlock Deck
Unlock for access to all 7 flashcards in this deck.
Unlock Deck
k this deck