Deck 8: User-Defined Classes and Adts
Question
Question
Question
Question
Question
Question
Question
Question
Question
Question
Question
Question
Question
Question
Question
Question
Question
Question
Question
Question
Question
Question
Question
Question
Question
Question
Question
Question
Question
Question
Question
Question
Question
Question
Question
Question
Question
Question
Question
Question
Question
Question
Question
Question
Question
Question
Question
Question
Question
Question
Unlock Deck
Sign up to unlock the cards in this deck!
Unlock Deck
Unlock Deck
1/50
Play
Full screen (f)
Deck 8: User-Defined Classes and Adts
1
An accessor method of a class first accesses the values of the data members of the class and then changes the values of the data members.
False
2
In deep copying, each reference variable refers to its own object.
True
3
Given the declaration public class MyClass {private int x; public void print() {System.out.println("x = " + x);} private void setX(int y) {x = y;}} MyClass myObject = new MyClass(); The following statement is legal. myObject.print();
True
4
When no object of the class type is instantiated, static data members of the class fail to exist.
Unlock Deck
Unlock for access to all 50 flashcards in this deck.
Unlock Deck
k this deck
5
Members of a class are usually classified into three categories: public, private, and static.
Unlock Deck
Unlock for access to all 50 flashcards in this deck.
Unlock Deck
k this deck
6
If the object is created in the definition of a method of the class, then the object can access both the public and private members of the class.
Unlock Deck
Unlock for access to all 50 flashcards in this deck.
Unlock Deck
k this deck
7
Not every user-defined class can override the default definition of the method toString because it is provided by Java.
Unlock Deck
Unlock for access to all 50 flashcards in this deck.
Unlock Deck
k this deck
8
The components of a class are called fields.
Unlock Deck
Unlock for access to all 50 flashcards in this deck.
Unlock Deck
k this deck
9
Constructors are called like any other method.
Unlock Deck
Unlock for access to all 50 flashcards in this deck.
Unlock Deck
k this deck
10
Modifiers are used to alter the behavior of the class.
Unlock Deck
Unlock for access to all 50 flashcards in this deck.
Unlock Deck
k this deck
11
The methods of a class must be public.
Unlock Deck
Unlock for access to all 50 flashcards in this deck.
Unlock Deck
k this deck
12
A constructor has no type and is therefore a void method.
Unlock Deck
Unlock for access to all 50 flashcards in this deck.
Unlock Deck
k this deck
13
If a member of a class is a private method, you can access it outside the class.
Unlock Deck
Unlock for access to all 50 flashcards in this deck.
Unlock Deck
k this deck
14
In shallow copying, each reference variable refers to its own object.
Unlock Deck
Unlock for access to all 50 flashcards in this deck.
Unlock Deck
k this deck
15
Given the declaration public class MyClass {private int x; public void print() {System.out.println("x = " + x);} private void setX(int y) {x = y;}} MyClass myObject = new MyClass(); The following statement is legal. myObject.setX(10);
Unlock Deck
Unlock for access to all 50 flashcards in this deck.
Unlock Deck
k this deck
16
The built-in operation that is valid for classes is the dot operator.
Unlock Deck
Unlock for access to all 50 flashcards in this deck.
Unlock Deck
k this deck
17
The non-static methods of a class are called instance methods.
Unlock Deck
Unlock for access to all 50 flashcards in this deck.
Unlock Deck
k this deck
18
The default constructor executes when an object is instantiated and initialized using an existing object.
Unlock Deck
Unlock for access to all 50 flashcards in this deck.
Unlock Deck
k this deck
19
The method finalize automatically executes when the class object goes out of scope.
Unlock Deck
Unlock for access to all 50 flashcards in this deck.
Unlock Deck
k this deck
20
A class and its members can be described graphically using Unified Modeling Language (UML) notation.
Unlock Deck
Unlock for access to all 50 flashcards in this deck.
Unlock Deck
k this deck
21
Which of the following is a constructor without parameters?
A) copy constructor
C) finalizer
B) default constructor
D) modifier
A) copy constructor
C) finalizer
B) default constructor
D) modifier
Unlock Deck
Unlock for access to all 50 flashcards in this deck.
Unlock Deck
k this deck
22
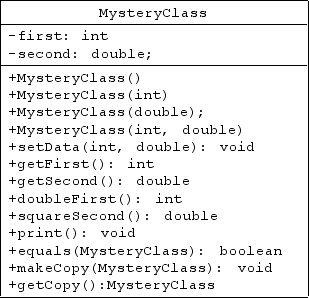
Which of the following would be a default constructor for the class MysteryClass shown in the accompanying figure?
A) public MysteryClass(){ setData(0, 0.0); }
B) public MysteryClass(0, 0.0) { setData(); }
C) public MysteryClass(0) { setData(0, 0.0); }
D) private MysteryClass(10){ setData(); }
Unlock Deck
Unlock for access to all 50 flashcards in this deck.
Unlock Deck
k this deck
23
Class members consist of all of the following EXCEPT ____.
A) named constants
C) pre-defined methods
B) variable declarations
D) methods
A) named constants
C) pre-defined methods
B) variable declarations
D) methods
Unlock Deck
Unlock for access to all 50 flashcards in this deck.
Unlock Deck
k this deck
24
The abstract data type specifies the logical properties and the implementation details.
Unlock Deck
Unlock for access to all 50 flashcards in this deck.
Unlock Deck
k this deck
25
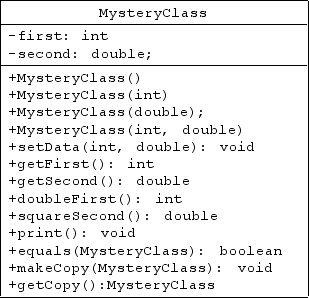
According to the UML class diagram in the accompanying figure, which method is public and doesn't return anything?
A) getCopy()
C) equals(MysteryClass)
B) print()
D) doubleFirst()
Unlock Deck
Unlock for access to all 50 flashcards in this deck.
Unlock Deck
k this deck
26
In Java, the reference this is used to refer to only the methods, not the instance variables of a class.
Unlock Deck
Unlock for access to all 50 flashcards in this deck.
Unlock Deck
k this deck
27
Constructors have the same name as the ____.
A) member methods
C) class
B) data members
D) package
A) member methods
C) class
B) data members
D) package
Unlock Deck
Unlock for access to all 50 flashcards in this deck.
Unlock Deck
k this deck
28
Consider the following class definition. public class Cylinder {private double baseRadius; private double height; public Cylinder () {baseRadius = 0; height = 0;} public Cylinder (double l, double h) {baseRadius = l; height = h;} public void set(double r, double h) {baseRadius = r; height = h;} public String toString() {return (baseRadius + " " + height);} public double SurfaceArea() {return 2 * 3.14 * baseRadius * height;} public double volume() {return 3.14 * baseRadius * baseRadius * height;}} Suppose that you have the following declaration. Cylinder cyl = new Cylinder(1.5, 10); Which of the following sets of statements are valid in Java? (i) cyl.surfaceArea(); cyl.volume(); cyl.print(); (ii) print(cyl.surfaceArea); print(cyl.volume());
A) Only (i)
C) Both (i) and (ii)
B) Only (ii)
D) None of these
A) Only (i)
C) Both (i) and (ii)
B) Only (ii)
D) None of these
Unlock Deck
Unlock for access to all 50 flashcards in this deck.
Unlock Deck
k this deck
29
A mutator method of a class changes the values of the data members of the class.
Unlock Deck
Unlock for access to all 50 flashcards in this deck.
Unlock Deck
k this deck
30
Which of the following class definitions is correct in Java? (i) public class Employee {private String name; private double salary; private int id; public Employee() {name = ""; salary = 0.0; id = 0;} public Employee(String n, double s, int i) {name = n; salary = s; id = i;} public void print() {System.out.println(name + " " + id + " " + salary);}} (ii) public class Employee {private String name; private double salary; private int id; public void Employee() {name = ""; salary = 0.0; id = 0;} public void Employee(String n, double s, int i) {name = n; salary = s; id = i;} public void print() {System.out.println(name + " " + id + " " + salary);}}
A) Only (i)
C) Both (i) and (ii)
B) Only (ii)
D) Neither is correct
A) Only (i)
C) Both (i) and (ii)
B) Only (ii)
D) Neither is correct
Unlock Deck
Unlock for access to all 50 flashcards in this deck.
Unlock Deck
k this deck
31
In ____ copying, each reference variable refers to its own object.
A) shallow
C) method
B) deep
D) object
A) shallow
C) method
B) deep
D) object
Unlock Deck
Unlock for access to all 50 flashcards in this deck.
Unlock Deck
k this deck
32
Consider the following statements. public class Circle {private double radius; public Circle() {radius = 0.0;} public Circle(double r) {radius = r;} public void set(double r) {radius = r;} public void print() {System.out.println(radius + " " + area + " " + circumference);} public double area() {return 3.14 * radius * radius;} public double circumference() {return 2 * 3.14 * radius;}} Circle myCircle = new Circle(); double r; Which of the following statements are valid in Java? (Assume that console is Scanner object initialized to the standard input device.) (i) r = console.nextDouble(); myCircle.area = 3.14 * r * r; System.out.println(myCircle.area); (ii) r = console.nextDouble(); myCircle.set(r); System.out.println(myCircle.area());
A) Only (i)
C) Both (i) and (ii)
B) Only (ii)
D) None of these
A) Only (i)
C) Both (i) and (ii)
B) Only (ii)
D) None of these
Unlock Deck
Unlock for access to all 50 flashcards in this deck.
Unlock Deck
k this deck
33
Consider the following class definition. public class Rectangle {private double length; private double width; public Rectangle() {length = 0; width = 0;} public Rectangle(double l, double w) {length = l; width = w;} public void set(double l, double w) {length = l; width = w;} public void print() {System.out.println(length + " " + width);} public double area() {return length * width;} public double perimeter() {return 2 * length + 2 * width;}} Which of the following statements correctly instantiates the Rectangle object myRectangle? (i) myRectangle = new Rectangle(12.5, 6); (ii) Rectangle myRectangle = new Rectangle(12.5, 6); (iii) class Rectangle myRectangle = new Rectangle(12.5, 6);
A) Only (i)
C) Only (iii)
B) Only (ii)
D) Both (ii) and (iii)
A) Only (i)
C) Only (iii)
B) Only (ii)
D) Both (ii) and (iii)
Unlock Deck
Unlock for access to all 50 flashcards in this deck.
Unlock Deck
k this deck
34
Which of the following is used to allocate memory for the instance variables of an object of a class?
A) the reserved word public
C) the operator new
B) the reserved word static
D) the operator +
A) the reserved word public
C) the operator new
B) the reserved word static
D) the operator +
Unlock Deck
Unlock for access to all 50 flashcards in this deck.
Unlock Deck
k this deck
35
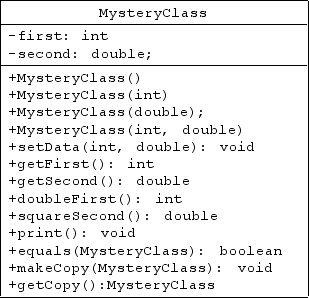
According to the UML class diagram in the accompanying figure, which of the following is a private member of the class MysteryClass?
A) doubleFirst
C) second
B) MysteryClass
D) print
Unlock Deck
Unlock for access to all 50 flashcards in this deck.
Unlock Deck
k this deck
36
Consider the following statements. public class Rectangle {private double length; private double width; public Rectangle() {length = 0; width = 0;} public Rectangle(double l, double w) {length = l; width = w;} public void set(double l, double w) {length = l; width = w;} public void print() {System.out.println("Length = " + length + "; Width = " + width + "\n" + + " Area = " + area() + "; Perimeter = " + perimeter());} public double area() {return length * width;} public void perimeter() {return 2 * length + 2 * width;} public void makeCopy(Rectangle otherRect) {length = otherRect.length; width = otherRect.width}} Rectangle tempRect = new Rectangle(14, 10); Rectangle newRect = new Rectangle(9, 5); What are the values of the instance variables of newRect after the following statement execute? newRect.makeCopy(tempRect);
A) length = 14; width = 10
B) length = 9; width = 5
C) length = 14; width = 5
D) None of these
A) length = 14; width = 10
B) length = 9; width = 5
C) length = 14; width = 5
D) None of these
Unlock Deck
Unlock for access to all 50 flashcards in this deck.
Unlock Deck
k this deck
37
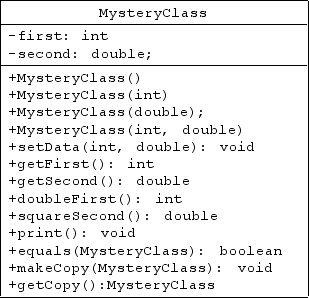
According to the UML class diagram in the accompanying figure, which of the following is a data member?
A) MysteryClass
C) second
B) print()
D) getFirst
Unlock Deck
Unlock for access to all 50 flashcards in this deck.
Unlock Deck
k this deck
38
Every object has access to a reference to itself.
Unlock Deck
Unlock for access to all 50 flashcards in this deck.
Unlock Deck
k this deck
39
What is the default definition of the method toString?
A) There is no default definition; you must define the method yourself.
B) It creates a string that is the name of the object's class, followed by the hash code of the object.
C) It creates a string that is the name of the program.
D) It creates a string that is the name of the package, followed by the name of the class, followed by the hash of the object.
A) There is no default definition; you must define the method yourself.
B) It creates a string that is the name of the object's class, followed by the hash code of the object.
C) It creates a string that is the name of the program.
D) It creates a string that is the name of the package, followed by the name of the class, followed by the hash of the object.
Unlock Deck
Unlock for access to all 50 flashcards in this deck.
Unlock Deck
k this deck
40
Classes that are defined within other classes are called inside classes.
Unlock Deck
Unlock for access to all 50 flashcards in this deck.
Unlock Deck
k this deck
41
Which of the following words indicates an object's reference to itself?
A) this
C) public
B) that
D) protected
A) this
C) public
B) that
D) protected
Unlock Deck
Unlock for access to all 50 flashcards in this deck.
Unlock Deck
k this deck
42
How many constructors are present in the class definition in the accompanying figure?
A) zero
C) two
B) one
D) three
A) zero
C) two
B) one
D) three
Unlock Deck
Unlock for access to all 50 flashcards in this deck.
Unlock Deck
k this deck
43
What does the default constructor do in the class definition in the accompanying figure?
A) Sets the value of x to 0
B) Sets the value of z to 1
C) Sets the value of x to 0 and the value of z to 1
D) There is no default constructor.
A) Sets the value of x to 0
B) Sets the value of z to 1
C) Sets the value of x to 0 and the value of z to 1
D) There is no default constructor.
Unlock Deck
Unlock for access to all 50 flashcards in this deck.
Unlock Deck
k this deck
44
What is the main use of inner classes?
A) To alter the behavior of the outer class
B) To handle events
C) To implement cascaded method calls
D) To provide information hiding
A) To alter the behavior of the outer class
B) To handle events
C) To implement cascaded method calls
D) To provide information hiding
Unlock Deck
Unlock for access to all 50 flashcards in this deck.
Unlock Deck
k this deck
45
How many finalizers can a class have?
A) zero
C) two
B) one
D) Any number
A) zero
C) two
B) one
D) Any number
Unlock Deck
Unlock for access to all 50 flashcards in this deck.
Unlock Deck
k this deck
46
Based on the class in the accompanying figure, which of the following statements is illegal?
A) Secret.incrementY();
B) Secret.count++;
C) Secret.z++;
D) Secret secret = new Secret(4);
A) Secret.incrementY();
B) Secret.count++;
C) Secret.z++;
D) Secret secret = new Secret(4);
Unlock Deck
Unlock for access to all 50 flashcards in this deck.
Unlock Deck
k this deck
47
ADTs illustrate the principle of ____.
A) information hiding
C) encapsulation
B) privacy
D) overloading
A) information hiding
C) encapsulation
B) privacy
D) overloading
Unlock Deck
Unlock for access to all 50 flashcards in this deck.
Unlock Deck
k this deck
48
When does the method finalize execute?
A) When the class object is created
B) At the start of every program
C) At the end of every program
D) When the class object goes out of scope
A) When the class object is created
B) At the start of every program
C) At the end of every program
D) When the class object goes out of scope
Unlock Deck
Unlock for access to all 50 flashcards in this deck.
Unlock Deck
k this deck
49
What might the heading of the copy constructor for the class in the accompanying figure look like?
A) public Secret(Secret s)
B) private copySecret()
C) Secret copySecret = new Secret()
D) public copySecret(int a, int b)
A) public Secret(Secret s)
B) private copySecret()
C) Secret copySecret = new Secret()
D) public copySecret(int a, int b)
Unlock Deck
Unlock for access to all 50 flashcards in this deck.
Unlock Deck
k this deck
50
Suppose that Book is class with two instance variables-name of type String and numOfBooks of type int. Which of the following would be appropriate syntax for the heading of a copy constructor for the class called Book?
A) public Book(String n, int num)
B) public Book()
C) public Book(Book b)
D) public copyBook(String n, int num)
A) public Book(String n, int num)
B) public Book()
C) public Book(Book b)
D) public copyBook(String n, int num)
Unlock Deck
Unlock for access to all 50 flashcards in this deck.
Unlock Deck
k this deck