Deck 12: Arrays Continued
Question
Question
Question
Question
Question
Question
Question
Question
Question
Question
Question
Question
Question
Question
Question
Question
Question
Question
Question
Question
Question
Question
Question
Question
Question
Question
Question
Question
Question
Question
Question
Question
Question
Question
Question
Question
Question
Question
Question
Question
Question
Question
Question
Question
Question
Question
Question
Unlock Deck
Sign up to unlock the cards in this deck!
Unlock Deck
Unlock Deck
1/47
Play
Full screen (f)
Deck 12: Arrays Continued
1
Each sort method and its helper methods should be defined as private static. ____________________
True
2
When inserting an item into an array, assume that ____.
A) 0 <= target index = logical size
B) 0 < target index <= logical size
C) 0 <= target index < logical size
D) 0 <= target index <= logical size
A) 0 <= target index = logical size
B) 0 < target index <= logical size
C) 0 <= target index < logical size
D) 0 <= target index <= logical size
D
3
A(n) ____ search examines every element to determine the absence of a target.
A) linear
B) binary
C) bubble
D) selection
A) linear
B) binary
C) bubble
D) selection
A
4
Selection, bubble, and insertion are simple sort methods that work well for small- and medium-sized arrays.
Unlock Deck
Unlock for access to all 47 flashcards in this deck.
Unlock Deck
k this deck
5
One example of a two-dimensional grid is a screen coordinate system for a graphics application.
Unlock Deck
Unlock for access to all 47 flashcards in this deck.
Unlock Deck
k this deck
6
____ is the process of arranging array elements.
A) Searching
B) Sorting
C) Selecting
D) Ordering
A) Searching
B) Sorting
C) Selecting
D) Ordering
Unlock Deck
Unlock for access to all 47 flashcards in this deck.
Unlock Deck
k this deck
7
The selection sort will not allow an automatic loop exit if the array becomes ordered during an early pass.
Unlock Deck
Unlock for access to all 47 flashcards in this deck.
Unlock Deck
k this deck
8
You should test sort methods with an array that is already sorted.
Unlock Deck
Unlock for access to all 47 flashcards in this deck.
Unlock Deck
k this deck
9
The basic idea of an insertion sort is that for each index position (i), find the smallest data value in the positions i through length -1, then exchange the smallest value with the value at position i. ____________________
Unlock Deck
Unlock for access to all 47 flashcards in this deck.
Unlock Deck
k this deck
10
When removing an item from an array, you must decrement the physical size by one. ____________________
Unlock Deck
Unlock for access to all 47 flashcards in this deck.
Unlock Deck
k this deck
11
A linear search works well for arrays that are very large (thousands or millions of elements).
Unlock Deck
Unlock for access to all 47 flashcards in this deck.
Unlock Deck
k this deck
12
A(n) ____ sort causes a pass through the array to compare adjacent pairs of items.
A) selection
B) insertion
C) bubble
D) binary
A) selection
B) insertion
C) bubble
D) binary
Unlock Deck
Unlock for access to all 47 flashcards in this deck.
Unlock Deck
k this deck
13
When replacing an item in an array, the logical size changes.
Unlock Deck
Unlock for access to all 47 flashcards in this deck.
Unlock Deck
k this deck
14
In a linear search, the loop breaks if the target is found. ____________________
Unlock Deck
Unlock for access to all 47 flashcards in this deck.
Unlock Deck
k this deck
15
When inserting and removing items from an array, the order in which items are shifted is not important.
Unlock Deck
Unlock for access to all 47 flashcards in this deck.
Unlock Deck
k this deck
16
A drop-down menu is used to hide commands under visible labels to use GUI window space efficiently. ____________________
Unlock Deck
Unlock for access to all 47 flashcards in this deck.
Unlock Deck
k this deck
17
The elements of a two-dimensional array can be of two different types.
Unlock Deck
Unlock for access to all 47 flashcards in this deck.
Unlock Deck
k this deck
18
A(n) ____ search starts at the middle of an array.
A) linear
B) binary
C) bubble
D) selection
A) linear
B) binary
C) bubble
D) selection
Unlock Deck
Unlock for access to all 47 flashcards in this deck.
Unlock Deck
k this deck
19
Objects can be compared using the < and > operators.
Unlock Deck
Unlock for access to all 47 flashcards in this deck.
Unlock Deck
k this deck
20
The Object class implements the Comparable interface.
Unlock Deck
Unlock for access to all 47 flashcards in this deck.
Unlock Deck
k this deck
21
When removing an item from an array, assume that ____.
A) 0 <= target index = logical size
B) 0 < target index <= logical size
C) 0 <= target index < logical size
D) 0 <= target index <= logical size
A) 0 <= target index = logical size
B) 0 < target index <= logical size
C) 0 <= target index < logical size
D) 0 <= target index <= logical size
Unlock Deck
Unlock for access to all 47 flashcards in this deck.
Unlock Deck
k this deck
22
David knows that when removing an element, the target index needs to be ____ the logical size
A) less than
B) less than or equal to
C) equal to
D) greater than or equal to
A) less than
B) less than or equal to
C) equal to
D) greater than or equal to
Unlock Deck
Unlock for access to all 47 flashcards in this deck.
Unlock Deck
k this deck
23
When inserting an item from an array, if there is not room in the array, ____.
A) false is returned
B) the operation is not performed
C) the physical size of the array is increased
D) both a and b are true
A) false is returned
B) the operation is not performed
C) the physical size of the array is increased
D) both a and b are true
Unlock Deck
Unlock for access to all 47 flashcards in this deck.
Unlock Deck
k this deck
24
When creating a drop-down menu, each menu item needs to have a(n) ____ attached to it.
A) panel
B) container
C) widget
D) listener
A) panel
B) container
C) widget
D) listener
Unlock Deck
Unlock for access to all 47 flashcards in this deck.
Unlock Deck
k this deck
25
FIGURE 12-1 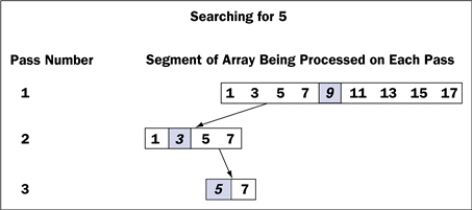
Figure 12-1 above shows a trace of a(n) ____ search.
A) linear
B) binary
C) bubble
D) selection
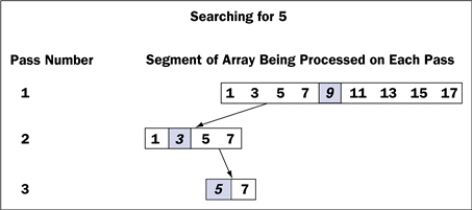
Figure 12-1 above shows a trace of a(n) ____ search.
A) linear
B) binary
C) bubble
D) selection
Unlock Deck
Unlock for access to all 47 flashcards in this deck.
Unlock Deck
k this deck
26
When comparing two string elements, the ____ must be used.
A) = = operator
B) equals method
C) compareString method
D) selection sort
A) = = operator
B) equals method
C) compareString method
D) selection sort
Unlock Deck
Unlock for access to all 47 flashcards in this deck.
Unlock Deck
k this deck
27
FIGURE 12-2 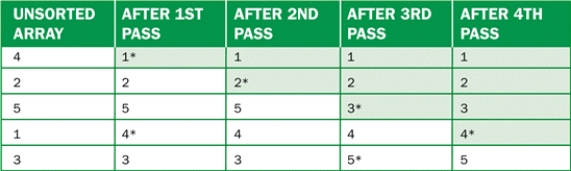
David knows that when inserting an element, the target index needs to be ____ the logical size
A) less than
B) less than or equal to
C) equal to
D) greater than or equal to
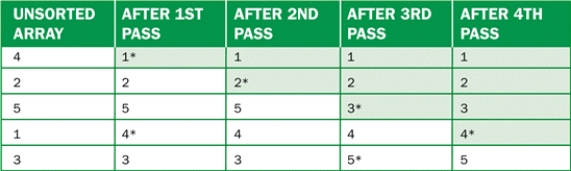
David knows that when inserting an element, the target index needs to be ____ the logical size
A) less than
B) less than or equal to
C) equal to
D) greater than or equal to
Unlock Deck
Unlock for access to all 47 flashcards in this deck.
Unlock Deck
k this deck
28
FIGURE 12-2 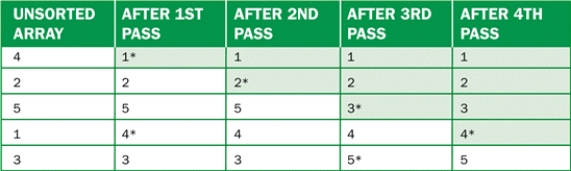
Figure 12-2 above shows an example of a trace of data during a(n) ____ sort.
A) insertion
B) bubble
C) linear
D) selection
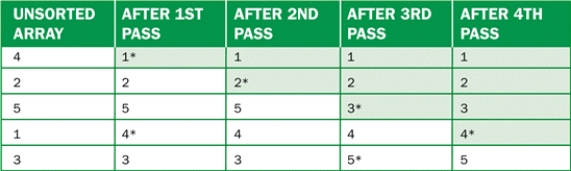
Figure 12-2 above shows an example of a trace of data during a(n) ____ sort.
A) insertion
B) bubble
C) linear
D) selection
Unlock Deck
Unlock for access to all 47 flashcards in this deck.
Unlock Deck
k this deck
29
In a(n) ____________________ search, the number of elements yet to be examined is reduced by half.
Unlock Deck
Unlock for access to all 47 flashcards in this deck.
Unlock Deck
k this deck
30
Which of the following is NOT true about algorithms for a selection sort?
A) The <= inequality is used.
B) If the array is of length n, you need n-1 steps.
C) You must be able to find the smallest number.
D) You need to exchange appropriate array items.
A) The <= inequality is used.
B) If the array is of length n, you need n-1 steps.
C) You must be able to find the smallest number.
D) You need to exchange appropriate array items.
Unlock Deck
Unlock for access to all 47 flashcards in this deck.
Unlock Deck
k this deck
31
A(n) ____ search assumes the elements are sorted.
A) bubble
B) linear
C) binary
D) selection
A) bubble
B) linear
C) binary
D) selection
Unlock Deck
Unlock for access to all 47 flashcards in this deck.
Unlock Deck
k this deck
32
The bubble sort algorithm involves a(n) ____________________ loop structure.
Unlock Deck
Unlock for access to all 47 flashcards in this deck.
Unlock Deck
k this deck
33
To add a drop-down menu to a GUI, you need to add all of the following EXCEPT ____.
A) buttons
B) menu bar
C) menus
D) menu items
A) buttons
B) menu bar
C) menus
D) menu items
Unlock Deck
Unlock for access to all 47 flashcards in this deck.
Unlock Deck
k this deck
34
The ____ search is faster when searching an array of elements for a target element.
A) bubble
B) linear
C) binary
D) selection
A) bubble
B) linear
C) binary
D) selection
Unlock Deck
Unlock for access to all 47 flashcards in this deck.
Unlock Deck
k this deck
35
Using a(n) ____ list with a two-dimensional array requires a list of lists.
A) initializer
B) string
C) abstract
D) concrete
A) initializer
B) string
C) abstract
D) concrete
Unlock Deck
Unlock for access to all 47 flashcards in this deck.
Unlock Deck
k this deck
36
Before sending the compareTo message to an arbitrary object, the object must be cast to ____.
A) Object
B) Comparable
C) Evaluate
D) Search
A) Object
B) Comparable
C) Evaluate
D) Search
Unlock Deck
Unlock for access to all 47 flashcards in this deck.
Unlock Deck
k this deck
37
The method ____ can be used instead of <, >, and = =.
A) moreLessEqual
B) evaluate
C) Comparable
D) compareTo
A) moreLessEqual
B) evaluate
C) Comparable
D) compareTo
Unlock Deck
Unlock for access to all 47 flashcards in this deck.
Unlock Deck
k this deck
38
When inserting an item into an array, you must shift the items from the target index to the ____________________ end of the array up by one position.
Unlock Deck
Unlock for access to all 47 flashcards in this deck.
Unlock Deck
k this deck
39
For each pass of a(n) ____ search, the current leftmost position or current rightmost position is adjusted to track the portion of the array being searched.
A) bubble
B) insertion
C) binary
D) linear
A) bubble
B) insertion
C) binary
D) linear
Unlock Deck
Unlock for access to all 47 flashcards in this deck.
Unlock Deck
k this deck
40
When inserting an item in an array, start at the ____.
A) physical end of the array
B) logical end of the array
C) middle of the array
D) 0 position of the array
A) physical end of the array
B) logical end of the array
C) middle of the array
D) 0 position of the array
Unlock Deck
Unlock for access to all 47 flashcards in this deck.
Unlock Deck
k this deck
41
In a(n) ____________________ array, the rows are not all the same length.
Unlock Deck
Unlock for access to all 47 flashcards in this deck.
Unlock Deck
k this deck
42
Identify the letter of the choice that best matches the phrase or definition.
a.Logical size
b.Two-dimensional array
c.Ragged array
d.Tic-tac-toe game
e.Drop-down menu
Must include elements all of the same type.
a.Logical size
b.Two-dimensional array
c.Ragged array
d.Tic-tac-toe game
e.Drop-down menu
Must include elements all of the same type.
Unlock Deck
Unlock for access to all 47 flashcards in this deck.
Unlock Deck
k this deck
43
Identify the letter of the choice that best matches the phrase or definition.
a.Logical size
b.Two-dimensional array
c.Ragged array
d.Tic-tac-toe game
e.Drop-down menu
Is decremented by one when removing an item from an array.
a.Logical size
b.Two-dimensional array
c.Ragged array
d.Tic-tac-toe game
e.Drop-down menu
Is decremented by one when removing an item from an array.
Unlock Deck
Unlock for access to all 47 flashcards in this deck.
Unlock Deck
k this deck
44
A(n) ____________________ search works well for elements that are in ascending order.
Unlock Deck
Unlock for access to all 47 flashcards in this deck.
Unlock Deck
k this deck
45
Identify the letter of the choice that best matches the phrase or definition.
a.Logical size
b.Two-dimensional array
c.Ragged array
d.Tic-tac-toe game
e.Drop-down menu
Used to combine commands in a GUI window.
a.Logical size
b.Two-dimensional array
c.Ragged array
d.Tic-tac-toe game
e.Drop-down menu
Used to combine commands in a GUI window.
Unlock Deck
Unlock for access to all 47 flashcards in this deck.
Unlock Deck
k this deck
46
Identify the letter of the choice that best matches the phrase or definition.
a.Logical size
b.Two-dimensional array
c.Ragged array
d.Tic-tac-toe game
e.Drop-down menu
An example is a table where the first row as 6 elements and the second row has 10 elements.
a.Logical size
b.Two-dimensional array
c.Ragged array
d.Tic-tac-toe game
e.Drop-down menu
An example is a table where the first row as 6 elements and the second row has 10 elements.
Unlock Deck
Unlock for access to all 47 flashcards in this deck.
Unlock Deck
k this deck
47
Identify the letter of the choice that best matches the phrase or definition.
a.Logical size
b.Two-dimensional array
c.Ragged array
d.Tic-tac-toe game
e.Drop-down menu
An example of a two-dimensional grid.
a.Logical size
b.Two-dimensional array
c.Ragged array
d.Tic-tac-toe game
e.Drop-down menu
An example of a two-dimensional grid.
Unlock Deck
Unlock for access to all 47 flashcards in this deck.
Unlock Deck
k this deck