Deck 5: Sequential Containers
Question
Question
Question
Question
Question
Question
Question
Question
Question
Question
Question
Question
Question
Question
Question
Question
Question
Question
Question
Question
Question
Question
Question
Question
Question
Unlock Deck
Sign up to unlock the cards in this deck!
Unlock Deck
Unlock Deck
1/25
Play
Full screen (f)
Deck 5: Sequential Containers
1
A template class is a class that stores and processes a collection of information.
True
2
If you remove an element from a vector object, the size automatically decreases.
True
3
The for loop below is designed to populate an int type vector named my_vector with the first ten odd positive integers. Using the vector class at() function, complete the for loop.
for (int index = 0; index ++; index < 10)
__________
for (int index = 0; index ++; index < 10)
__________
my_vector.at(index) = 2 * index + 1;
4
When implementing a(n) __________ class, all of the code must be in the header or in a file included by the header.
A) derived
B) base
C) public
D) template
A) derived
B) base
C) public
D) template
Unlock Deck
Unlock for access to all 25 flashcards in this deck.
Unlock Deck
k this deck
5
Which of the following headers changes the subscript operator defined below so that it returns a non-modifiable reference?
 B) const Item_Type& operator[](size_t index) const C) Item_Type& operator[](size_t index) const D) static Item_Type& operator[](size_t index) const](https://d2lvgg3v3hfg70.cloudfront.net/TB10563/11eec35c_d7df_ccc8_ae1f_477fa28f4307_TB10563_00.jpg)
A) const Item_Type& operator[](size_t index)
B) const Item_Type& operator[](size_t index) const
C) Item_Type& operator[](size_t index) const
D) static Item_Type& operator[](size_t index) const
 B) const Item_Type& operator[](size_t index) const C) Item_Type& operator[](size_t index) const D) static Item_Type& operator[](size_t index) const](https://d2lvgg3v3hfg70.cloudfront.net/TB10563/11eec35c_d7df_ccc8_ae1f_477fa28f4307_TB10563_00.jpg)
A) const Item_Type& operator[](size_t index)
B) const Item_Type& operator[](size_t index) const
C) Item_Type& operator[](size_t index) const
D) static Item_Type& operator[](size_t index) const
Unlock Deck
Unlock for access to all 25 flashcards in this deck.
Unlock Deck
k this deck
6
To insert an item into the middle of the array, the values that are at the __________ point and beyond must be shifted over to make room.
A) insertion
B) starting
C) mid
D) end
A) insertion
B) starting
C) mid
D) end
Unlock Deck
Unlock for access to all 25 flashcards in this deck.
Unlock Deck
k this deck
7
Most of the user-defined operational expense in the KW::vector class reserve function is attributable to the for loop excerpted below.
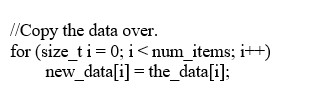
Using order of growth techniques against this code fragment, we can estimate that each reallocation of reserve () is __________.
A) O(1)
B) O(n log2n)
C) O(n)
D) O(n2)
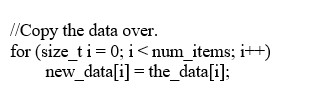
Using order of growth techniques against this code fragment, we can estimate that each reallocation of reserve () is __________.
A) O(1)
B) O(n log2n)
C) O(n)
D) O(n2)
Unlock Deck
Unlock for access to all 25 flashcards in this deck.
Unlock Deck
k this deck
8
Even if we have to reallocate in a call to KW::vector class insert (), the cost of the operation would still be O(__________).
Unlock Deck
Unlock for access to all 25 flashcards in this deck.
Unlock Deck
k this deck
9
The purpose of the copy constructor is to create a shallow copy of an object.
Unlock Deck
Unlock for access to all 25 flashcards in this deck.
Unlock Deck
k this deck
10
For class types, assignment is done by what is appropriately known as the __________ operator.
Unlock Deck
Unlock for access to all 25 flashcards in this deck.
Unlock Deck
k this deck
11
The variable called "this" is a(n) __________ to the object to which a member function is applied.
Unlock Deck
Unlock for access to all 25 flashcards in this deck.
Unlock Deck
k this deck
12
A(n) __________ list provides the ability to add or remove items anywhere in the list in constant (O(1)) time.
Unlock Deck
Unlock for access to all 25 flashcards in this deck.
Unlock Deck
k this deck
13
A(n) __________ is a pointer to a node.
A) struct
B) node
C) link
D) item
A) struct
B) node
C) link
D) item
Unlock Deck
Unlock for access to all 25 flashcards in this deck.
Unlock Deck
k this deck
14
Provide a critical line of code that will enable the while loop below to terminate.
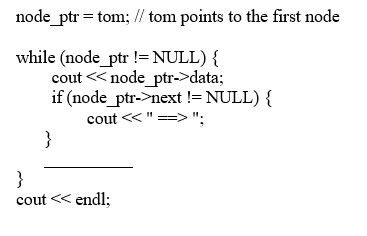
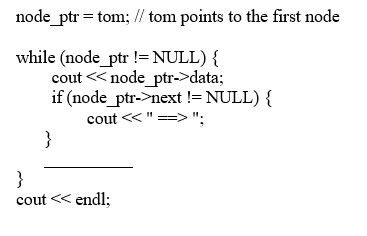
Unlock Deck
Unlock for access to all 25 flashcards in this deck.
Unlock Deck
k this deck
15
You can create a __________ liked list by linking the last node to the first node (and the first node to the last one).
A) single
B) circular
C) double
D) reverse
A) single
B) circular
C) double
D) reverse
Unlock Deck
Unlock for access to all 25 flashcards in this deck.
Unlock Deck
k this deck
16
A(n) __________ is a moving place marker that can be advanced forward (using operator++) or backward (using operator--).
Unlock Deck
Unlock for access to all 25 flashcards in this deck.
Unlock Deck
k this deck
17
In the context of iterators, a(n) __________ represents a common interface that a generic class must meet.
Unlock Deck
Unlock for access to all 25 flashcards in this deck.
Unlock Deck
k this deck
18
What value is output after the final line in the following code segment executes?
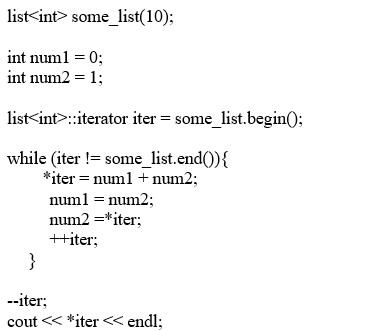
A) -842150451
B) 0
C) 55
D) 89
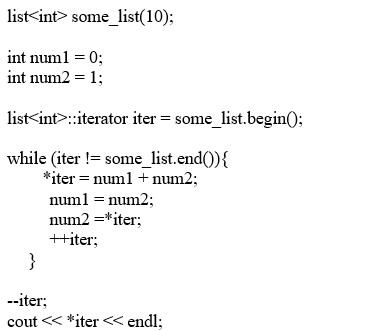
A) -842150451
B) 0
C) 55
D) 89
Unlock Deck
Unlock for access to all 25 flashcards in this deck.
Unlock Deck
k this deck
19
Which of the following conditions will enable this KW::list function to perform its task?
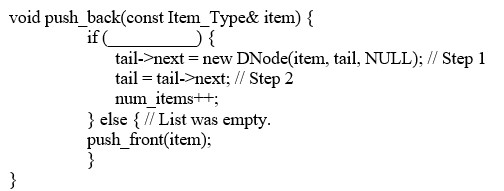
A) tail->next != NULL
B) tail != NULL
C) tail == NULL
D) tail->next == NULL
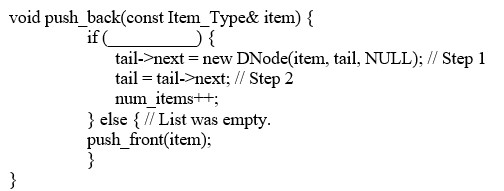
A) tail->next != NULL
B) tail != NULL
C) tail == NULL
D) tail->next == NULL
Unlock Deck
Unlock for access to all 25 flashcards in this deck.
Unlock Deck
k this deck
20
Which line will properly complete the definition for KW::iterator class postfix increment operator?
Iterator operator++(int) {
Iterator return_value = *this;
__________
Return return_value;
}
A) this++
B) ++this
C) --(*this)
D) ++(*this)
Iterator operator++(int) {
Iterator return_value = *this;
__________
Return return_value;
}
A) this++
B) ++this
C) --(*this)
D) ++(*this)
Unlock Deck
Unlock for access to all 25 flashcards in this deck.
Unlock Deck
k this deck
21
Because a list and a vector have several member functions in common, they may be used polymorphically.
Unlock Deck
Unlock for access to all 25 flashcards in this deck.
Unlock Deck
k this deck
22
The associative containers are designed for fast retrieval of data based on a unique value stored in each item, called its __________.
Unlock Deck
Unlock for access to all 25 flashcards in this deck.
Unlock Deck
k this deck
23
Provide the code that completes the definition of the STL template find function below.
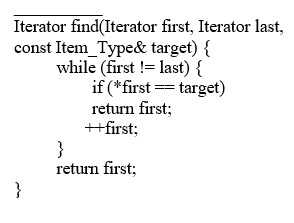
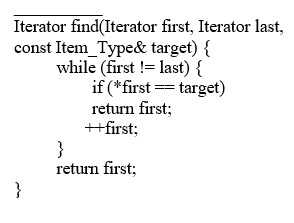
Unlock Deck
Unlock for access to all 25 flashcards in this deck.
Unlock Deck
k this deck
24
The template functions, or __________perform fairly standard operations on containers, such as applying the same function to each element (for_each), copying values from one container to another (copy), searching a container for a target value (find, find_if), or sorting a container (sort).
A) algorithms
B) procedures
C) methods
D) routines
A) algorithms
B) procedures
C) methods
D) routines
Unlock Deck
Unlock for access to all 25 flashcards in this deck.
Unlock Deck
k this deck
25
The function call operator cannot be overloaded by a class.
Unlock Deck
Unlock for access to all 25 flashcards in this deck.
Unlock Deck
k this deck