Deck 9: Records Structs
Question
Question
Question
Question
Question
Question
Question
Question
Question
Question
Question
Question
Question
Question
Question
Question
Question
Question
Question
Question
Question
Question
Question
Question
Question
Question
Question
Question
Question
Question
Question
Question
Question
Question
Question
Question
Question
Question
Question
Question
Unlock Deck
Sign up to unlock the cards in this deck!
Unlock Deck
Unlock Deck
1/40
Play
Full screen (f)
Deck 9: Records Structs
1
In structs, you access a component by using the struct name together with the relative position of the component.
False
2
The components of a struct are called the ____ of the struct.
A) variables
B) identifiers
C) elements
D) members
A) variables
B) identifiers
C) elements
D) members
D
3
Consider the following statements:
rectangleData bigRect; Which of the following statements is valid in C++?
A) cin >> bigRect;
B) cin >> bigRect.length;
C) perimeter = 2 * (length + width);
D) area = length * width;
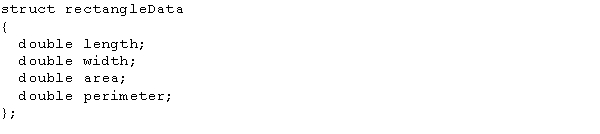
A) cin >> bigRect;
B) cin >> bigRect.length;
C) perimeter = 2 * (length + width);
D) area = length * width;
B
4
An array name and index are separated using ____.
A) curly brackets
B) square brackets
C) a dot
D) a comma
A) curly brackets
B) square brackets
C) a dot
D) a comma
Unlock Deck
Unlock for access to all 40 flashcards in this deck.
Unlock Deck
k this deck
5
A struct is typically a ____ data structure.
A) simple
B) dynamic
C) heterogeneous
D) linked
A) simple
B) dynamic
C) heterogeneous
D) linked
Unlock Deck
Unlock for access to all 40 flashcards in this deck.
Unlock Deck
k this deck
6
Consider the following struct definition:Which of the following variable declarations is correct? 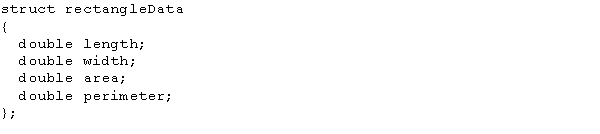
A) rectangle rectangleData;
B) struct rectangleData();
C) rectangleData myRectangle;
D) rectangleData rectangle = new rectangleData();
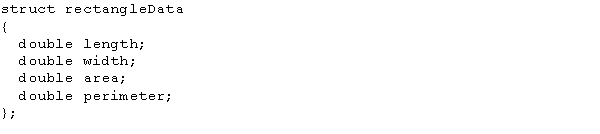
A) rectangle rectangleData;
B) struct rectangleData();
C) rectangleData myRectangle;
D) rectangleData rectangle = new rectangleData();
Unlock Deck
Unlock for access to all 40 flashcards in this deck.
Unlock Deck
k this deck
7
Typically, in a program, a struct is defined ____ in the program.
A) in the main function
B) before the definitions of all the functions
C) after the definitions of all the functions
D) in any function
A) in the main function
B) before the definitions of all the functions
C) after the definitions of all the functions
D) in any function
Unlock Deck
Unlock for access to all 40 flashcards in this deck.
Unlock Deck
k this deck
8
A function can return a value of the type array.
Unlock Deck
Unlock for access to all 40 flashcards in this deck.
Unlock Deck
k this deck
9
To access a structure member (component), you use the struct variable name together with the member name; these names are separated by a dot (period).
Unlock Deck
Unlock for access to all 40 flashcards in this deck.
Unlock Deck
k this deck
10
You can declare struct variables when you define a struct.
Unlock Deck
Unlock for access to all 40 flashcards in this deck.
Unlock Deck
k this deck
11
The syntax for accessing a struct member is structVariableName____.
A) .memberName
B) *memberName
C) [memberName]
D) $memberName
A) .memberName
B) *memberName
C) [memberName]
D) $memberName
Unlock Deck
Unlock for access to all 40 flashcards in this deck.
Unlock Deck
k this deck
12
Aggregate input/output operations are allowed on a struct variable.
Unlock Deck
Unlock for access to all 40 flashcards in this deck.
Unlock Deck
k this deck
13
Relational operations can be used on struct variables.
Unlock Deck
Unlock for access to all 40 flashcards in this deck.
Unlock Deck
k this deck
14
A function can return a value of the type struct.
Unlock Deck
Unlock for access to all 40 flashcards in this deck.
Unlock Deck
k this deck
15
Data in a struct variable must be read one member at a time.
Unlock Deck
Unlock for access to all 40 flashcards in this deck.
Unlock Deck
k this deck
16
Consider the following statements:rectangleData bigRect;
Which of the following statements correctly initializes the component length of bigRect?
A) bigRect = {10};
B) bigRect.length = 10;
C) length[0]= 10;
D) bigRect[0]= 10
![<strong>Consider the following statements:rectangleData bigRect; Which of the following statements correctly initializes the component length of bigRect?</strong> A) bigRect = {10}; B) bigRect.length = 10; C) length[0]= 10; D) bigRect[0]= 10](https://d2lvgg3v3hfg70.cloudfront.net/TB4785/11ea77fe_9b28_7617_bbd5_d1384803c64d_TB4785_00.jpg)
A) bigRect = {10};
B) bigRect.length = 10;
C) length[0]= 10;
D) bigRect[0]= 10
Unlock Deck
Unlock for access to all 40 flashcards in this deck.
Unlock Deck
k this deck
17
You can use an assignment statement to copy the contents of one struct into another struct of the same type.
Unlock Deck
Unlock for access to all 40 flashcards in this deck.
Unlock Deck
k this deck
18
In C++, the ____ symbol is an operator, called the member access operator.
A) :(colon)
B) .(dot)
C) ,(comma)
D) $ (dollar sign)
A) :(colon)
B) .(dot)
C) ,(comma)
D) $ (dollar sign)
Unlock Deck
Unlock for access to all 40 flashcards in this deck.
Unlock Deck
k this deck
19
Which of the following struct definitions is correct in C++? 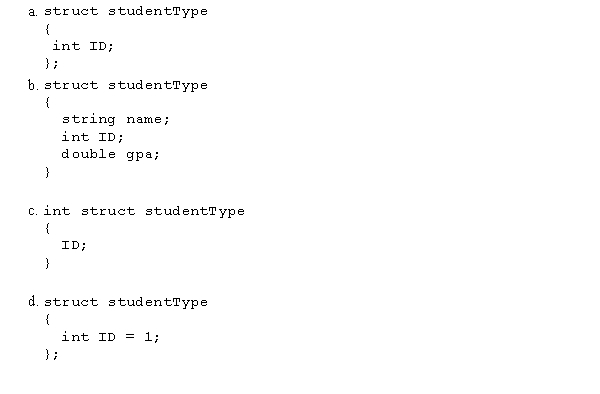
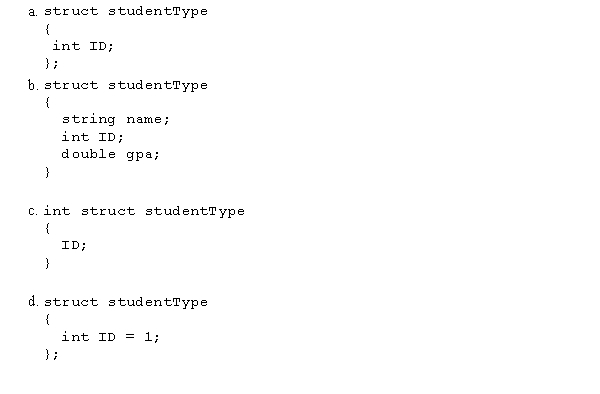
Unlock Deck
Unlock for access to all 40 flashcards in this deck.
Unlock Deck
k this deck
20
Consider the following statements:};
personalInfo person1, person2;
CommonInfo person3, person4;
Which of the following statements is valid in C++?
A) person1 = person3;
B) person2 = person1;
C) person2 = person3;
D) person2 = person4;
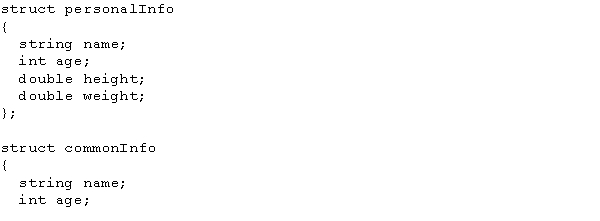
CommonInfo person3, person4;
Which of the following statements is valid in C++?
A) person1 = person3;
B) person2 = person1;
C) person2 = person3;
D) person2 = person4;
Unlock Deck
Unlock for access to all 40 flashcards in this deck.
Unlock Deck
k this deck
21
A struct is a(n) ____________________, not a declaration.
Unlock Deck
Unlock for access to all 40 flashcards in this deck.
Unlock Deck
k this deck
22
Consider the following statements:struct studentType1 studentType2 student3, student4;
Which of the following statements is valid in C++?
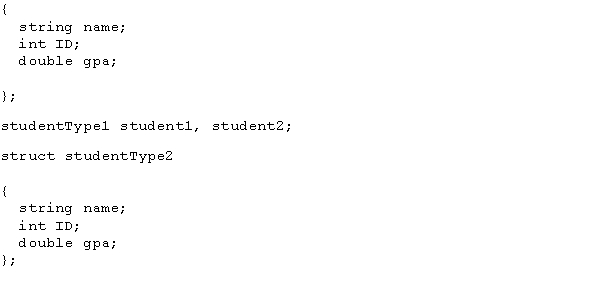
A) student2 = student3;
B) student1 = student4;
C) student2.ID = ID;
D) student1.ID = student3.ID;
Which of the following statements is valid in C++?
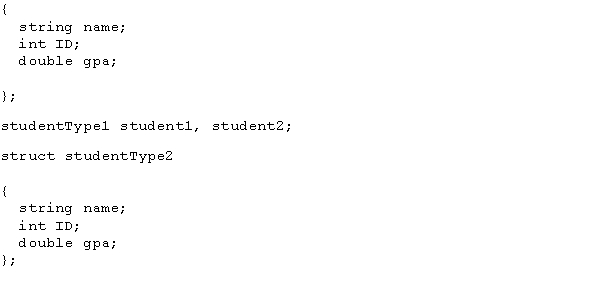
A) student2 = student3;
B) student1 = student4;
C) student2.ID = ID;
D) student1.ID = student3.ID;
Unlock Deck
Unlock for access to all 40 flashcards in this deck.
Unlock Deck
k this deck
23
To compare struct variables, you compare them ____.
A) by reference
B) by value
C) index-wise
D) member-wise
A) by reference
B) by value
C) index-wise
D) member-wise
Unlock Deck
Unlock for access to all 40 flashcards in this deck.
Unlock Deck
k this deck
24
A list has two items associated with it: ____.
A) the length and the references
B) the values and the references
C) the indexes and the length
D) the values and the length
A) the length and the references
B) the values and the references
C) the indexes and the length
D) the values and the length
Unlock Deck
Unlock for access to all 40 flashcards in this deck.
Unlock Deck
k this deck
25
Consider the following statements.
Which of the following statements is valid in C++?
A) cin >> circle.radius;
Circle.area = 3.14 * radius * radius;
B) cin >> circle.radius;
Circle.area = 3.14 * circle.radius * radius;
C) cin >> circle;
D) cin >> circle.radius;
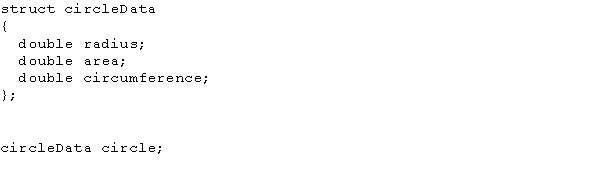
A) cin >> circle.radius;
Circle.area = 3.14 * radius * radius;
B) cin >> circle.radius;
Circle.area = 3.14 * circle.radius * radius;
C) cin >> circle;
D) cin >> circle.radius;
Unlock Deck
Unlock for access to all 40 flashcards in this deck.
Unlock Deck
k this deck
26
Consider the following statements:
rectangleData bigRect; rectangleData smallRect;
Which of the following statements is legal in C++?
A) if (bigRect == smallRect)
B) if (bigRect != smallRect)
C) if (bigRect.length == width)
D) if (bigRect.length == smallRect.width)
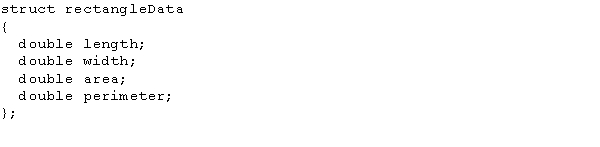
Which of the following statements is legal in C++?
A) if (bigRect == smallRect)
B) if (bigRect != smallRect)
C) if (bigRect.length == width)
D) if (bigRect.length == smallRect.width)
Unlock Deck
Unlock for access to all 40 flashcards in this deck.
Unlock Deck
k this deck
27
Consider the following statements:
Which of the following best describes applianceList?
A) It is a multidimensional array.
B) It is a struct.
C) It is an array of structs.
D) It is a struct of arrays.
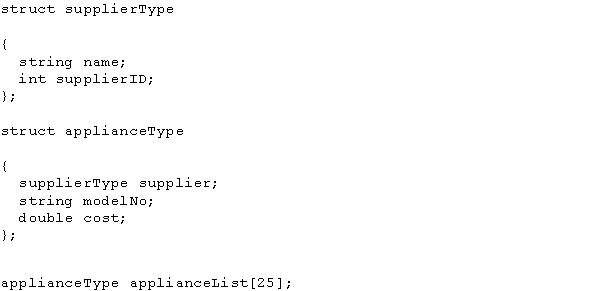
A) It is a multidimensional array.
B) It is a struct.
C) It is an array of structs.
D) It is a struct of arrays.
Unlock Deck
Unlock for access to all 40 flashcards in this deck.
Unlock Deck
k this deck
28
Arrays are passed by ____________________ only.
Unlock Deck
Unlock for access to all 40 flashcards in this deck.
Unlock Deck
k this deck
29
A struct variable can be passed as a parameter ____.
A) only by const
B) only by reference
C) only by value
D) either by value or by reference
A) only by const
B) only by reference
C) only by value
D) either by value or by reference
Unlock Deck
Unlock for access to all 40 flashcards in this deck.
Unlock Deck
k this deck
30
The following statement defines a struct houseType with a total of ____________________ member(s).
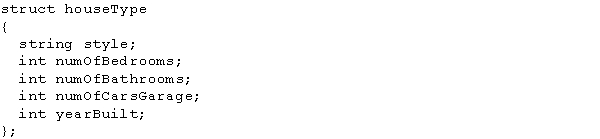
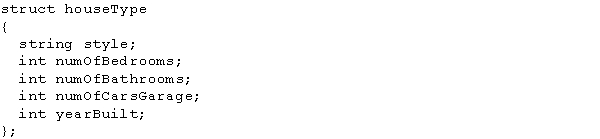
Unlock Deck
Unlock for access to all 40 flashcards in this deck.
Unlock Deck
k this deck
31
Which of the following is an allowable aggregate operation on a struct?
A) Arithmetic
B) Assignment
C) Input/output
D) Comparison
A) Arithmetic
B) Assignment
C) Input/output
D) Comparison
Unlock Deck
Unlock for access to all 40 flashcards in this deck.
Unlock Deck
k this deck
32
Memory is allocated for struct variables only when you ____________________ them.
Unlock Deck
Unlock for access to all 40 flashcards in this deck.
Unlock Deck
k this deck
33
Consider the following statements:
paintType paint; What is the data type of paint.supplier?
A) string
B) paintType
C) supplierType
D) struct
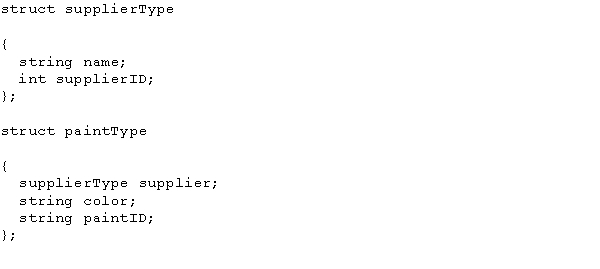
A) string
B) paintType
C) supplierType
D) struct
Unlock Deck
Unlock for access to all 40 flashcards in this deck.
Unlock Deck
k this deck
34
Which of the following aggregate operations can be executed on array variables?
A) Arithmetic
B) Assignment
C) Function returning a value
D) Parameter passing by reference
A) Arithmetic
B) Assignment
C) Function returning a value
D) Parameter passing by reference
Unlock Deck
Unlock for access to all 40 flashcards in this deck.
Unlock Deck
k this deck
35
You can assign the value of one struct variable to another struct variable of ____ type.
A) a simple data
B) the same
C) an array
D) any
A) a simple data
B) the same
C) an array
D) any
Unlock Deck
Unlock for access to all 40 flashcards in this deck.
Unlock Deck
k this deck
36
Consider the following function prototype:int seqSearch(const listType& list, int searchItem); The actual parameter cannot be modified by ____.
A) seqSearch
B) listType
C) list
D) searchItem
A) seqSearch
B) listType
C) list
D) searchItem
Unlock Deck
Unlock for access to all 40 flashcards in this deck.
Unlock Deck
k this deck
37
Consider the following statements:
rectangleData bigRect; Which of the following statements is valid in C++?
A) cin >> bigRect.length >> width;
B) cout << bigRect.length;
C) cout << bigRect;
D) cout << length;
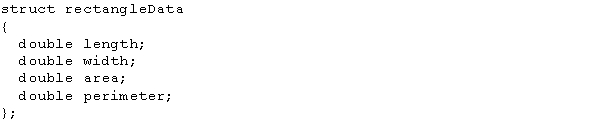
A) cin >> bigRect.length >> width;
B) cout << bigRect.length;
C) cout << bigRect;
D) cout << length;
Unlock Deck
Unlock for access to all 40 flashcards in this deck.
Unlock Deck
k this deck
38
If a variable is passed by ____________________, then when the formal parameter changes, the actual parameter also changes.
Unlock Deck
Unlock for access to all 40 flashcards in this deck.
Unlock Deck
k this deck
39
Consider the following statements:
applianceType applianceList[25]; Which of the following statements correctly initializes the cost of each appliance to 0?
A) applianceList.cost = 0;
B) applianceList.cost[25] = 0;
C) for (int j = 1; j < 25; j++)
ApplianceList.cost[j] = 0;
D) for (int j = 0; j < 25; j++)
ApplianceList.cost[j] = 0;
![<strong>Consider the following statements: applianceType applianceList[25]; Which of the following statements correctly initializes the cost of each appliance to 0?</strong> A) applianceList.cost = 0; B) applianceList.cost[25] = 0; C) for (int j = 1; j < 25; j++) ApplianceList.cost[j] = 0; D) for (int j = 0; j < 25; j++) ApplianceList.cost[j] = 0;](https://d2lvgg3v3hfg70.cloudfront.net/TB4785/11ea77fe_9b29_d5af_bbd5_9fe9fbb725a5_TB4785_00.jpg)
A) applianceList.cost = 0;
B) applianceList.cost[25] = 0;
C) for (int j = 1; j < 25; j++)
ApplianceList.cost[j] = 0;
D) for (int j = 0; j < 25; j++)
ApplianceList.cost[j] = 0;
Unlock Deck
Unlock for access to all 40 flashcards in this deck.
Unlock Deck
k this deck
40
Consider the following struct definition:The statement that declares intList to be a struct variable of type listType is ____________________.
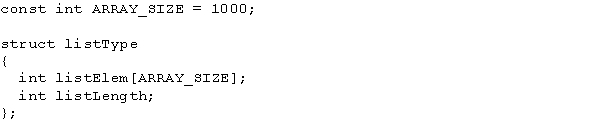
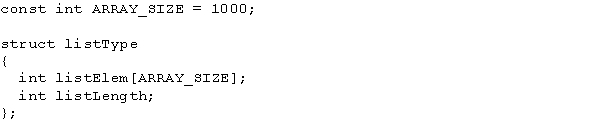
Unlock Deck
Unlock for access to all 40 flashcards in this deck.
Unlock Deck
k this deck