Deck 8: Arrays
Question
Question
Question
Question
Question
Question
Question
Question
Question
Question
Question
Question
Question
Question
Question
Question
Question
Question
Question
Question
Question
Question
Question
Question
Question
Question
Question
Question
Question
Question
Question
Question
Question
Question
Question
Question
Question
Question
Question
Question
Unlock Deck
Sign up to unlock the cards in this deck!
Unlock Deck
Unlock Deck
1/40
Play
Full screen (f)
Deck 8: Arrays
1
The one place where C++ allows aggregate operations on arrays is the input and output of C-strings.
True
2
Assume you have the following declaration char nameList[100];. Which of the following ranges is valid for the index of the array nameList?
A) 0 through 99
B) 0 through 100
C) 1 through 100
D) 1 through 101
A) 0 through 99
B) 0 through 100
C) 1 through 100
D) 1 through 101
A
3
Arrays can be passed as parameters to a function by value, but it is faster to pass them by reference.
False
4
What is the output of the following C++ code? 
A) 0 1 2 3 4
B) 0 5 10 15
C) 0 5 10 15 20
D) 5 10 15 20

A) 0 1 2 3 4
B) 0 5 10 15
C) 0 5 10 15 20
D) 5 10 15 20
Unlock Deck
Unlock for access to all 40 flashcards in this deck.
Unlock Deck
k this deck
5
The statement int list[25]; declares list to be an array of 26 components, since the array index starts at 0.
Unlock Deck
Unlock for access to all 40 flashcards in this deck.
Unlock Deck
k this deck
6
The array index can be any integer less than the array size.
Unlock Deck
Unlock for access to all 40 flashcards in this deck.
Unlock Deck
k this deck
7
Given the declaration int list[20]; the statement list[12] = list[5] + list[7]; updates the content of the twelfth component of the array list.
Unlock Deck
Unlock for access to all 40 flashcards in this deck.
Unlock Deck
k this deck
8
When you pass an array as a parameter, the base address of the actual array is passed to the formal parameter.
Unlock Deck
Unlock for access to all 40 flashcards in this deck.
Unlock Deck
k this deck
9
Suppose list is a one dimensional array of size 25, wherein each component is of type int. Further, suppose that sum is an int variable. The following for loop correctly finds the sum of the elements of list.


Unlock Deck
Unlock for access to all 40 flashcards in this deck.
Unlock Deck
k this deck
10
Suppose that sales is an array of 50 components of type double. Which of the following correctly initializes the array sales?
A) for (int 1 = 1; j <= 49; j++)
Sales[j] = 0;
B) for (int j = 1; j <= 50; j++)
Sales[j] = 0;
C) for (int j = 0; j <= 49; j++)
Sales[j] = 0.0;
D) for (int j = 0; j <= 50; j++)
Sales[j] = 0.0;
A) for (int 1 = 1; j <= 49; j++)
Sales[j] = 0;
B) for (int j = 1; j <= 50; j++)
Sales[j] = 0;
C) for (int j = 0; j <= 49; j++)
Sales[j] = 0.0;
D) for (int j = 0; j <= 50; j++)
Sales[j] = 0.0;
Unlock Deck
Unlock for access to all 40 flashcards in this deck.
Unlock Deck
k this deck
11
If an array index goes out of bounds, the program always terminates in an error.
Unlock Deck
Unlock for access to all 40 flashcards in this deck.
Unlock Deck
k this deck
12
What is the value of alpha[2] after the following code executes? ![<strong>What is the value of alpha[2] after the following code executes? </strong> A) 1 B) 4 C) 5 D) 6](https://d2lvgg3v3hfg70.cloudfront.net/TB4784/11ea7810_13f5_ffe5_bbd5_bba0112947b5_TB4784_00.jpg)
A) 1
B) 4
C) 5
D) 6
![<strong>What is the value of alpha[2] after the following code executes? </strong> A) 1 B) 4 C) 5 D) 6](https://d2lvgg3v3hfg70.cloudfront.net/TB4784/11ea7810_13f5_ffe5_bbd5_bba0112947b5_TB4784_00.jpg)
A) 1
B) 4
C) 5
D) 6
Unlock Deck
Unlock for access to all 40 flashcards in this deck.
Unlock Deck
k this deck
13
Which of the following statements declares alpha to be an array of 25 components of the type int?
A) int alpha[25];
B) int array alpha[25];
C) int alpha[2][5];
D) int array alpha[25][25];
A) int alpha[25];
B) int array alpha[25];
C) int alpha[2][5];
D) int array alpha[25][25];
Unlock Deck
Unlock for access to all 40 flashcards in this deck.
Unlock Deck
k this deck
14
In a two-dimensional array, the elements are arranged in a table form.
Unlock Deck
Unlock for access to all 40 flashcards in this deck.
Unlock Deck
k this deck
15
What is the output of the following C++ code? 
A) 0 5 10 15 20
B) 5 10 15 20 0
C) 5 10 15 20 20
D) Code results in index out-of-bounds

A) 0 5 10 15 20
B) 5 10 15 20 0
C) 5 10 15 20 20
D) Code results in index out-of-bounds
Unlock Deck
Unlock for access to all 40 flashcards in this deck.
Unlock Deck
k this deck
16
Assume you have the following declaration int beta[50];. Which of the following is a valid element of beta?
A) beta['2']
B) beta['50']
C) beta[0]
D) beta[50]
A) beta['2']
B) beta['50']
C) beta[0]
D) beta[50]
Unlock Deck
Unlock for access to all 40 flashcards in this deck.
Unlock Deck
k this deck
17
Suppose that list is an array of 10 components of type int. Which of the following codes correctly outputs all the elements of list?
A) for (int j = 1; j < 10; j++)
Cout << list[j] << " ";
Cout << endl;
B) for (int j = 0; j <= 9; j++)
Cout << list[j] << " ";
Cout << endl;
C) for (int j = 1; j < 11; j++)
Cout << list[j] << " ";
Cout << endl;
D) for (int j = 1; j <= 10; j++)
Cout << list[j] << " ";
Cout << endl;
A) for (int j = 1; j < 10; j++)
Cout << list[j] << " ";
Cout << endl;
B) for (int j = 0; j <= 9; j++)
Cout << list[j] << " ";
Cout << endl;
C) for (int j = 1; j < 11; j++)
Cout << list[j] << " ";
Cout << endl;
D) for (int j = 1; j <= 10; j++)
Cout << list[j] << " ";
Cout << endl;
Unlock Deck
Unlock for access to all 40 flashcards in this deck.
Unlock Deck
k this deck
18
What is the output of the following C++ code? 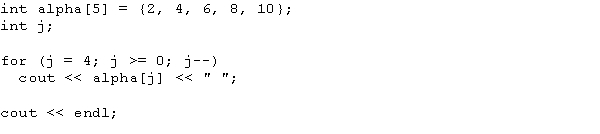
A) 2 4 6 8 10
B) 4 3 2 1 0
C) 8 6 4 2 0
D) 10 8 6 4 2
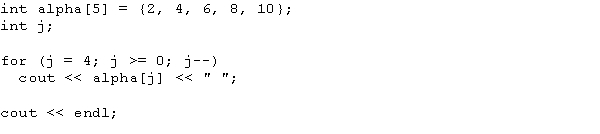
A) 2 4 6 8 10
B) 4 3 2 1 0
C) 8 6 4 2 0
D) 10 8 6 4 2
Unlock Deck
Unlock for access to all 40 flashcards in this deck.
Unlock Deck
k this deck
19
Assume you have the following declaration double salesData[1000];. Which of the following ranges is valid for the index of the array salesData?
A) 0 through 999
B) 0 through 1000
C) 1 through 1001
D) 1 through 1000
A) 0 through 999
B) 0 through 1000
C) 1 through 1001
D) 1 through 1000
Unlock Deck
Unlock for access to all 40 flashcards in this deck.
Unlock Deck
k this deck
20
All components of an array are of the same data type.
Unlock Deck
Unlock for access to all 40 flashcards in this deck.
Unlock Deck
k this deck
21
For a list of length n, the ____________________ sort makes exactly (n(n - 1))/2 key comparisons and 3(n - 1) item assignments.
Unlock Deck
Unlock for access to all 40 flashcards in this deck.
Unlock Deck
k this deck
22
The ____________________ of an array is the address (that is, the memory location) of the first array component.
Unlock Deck
Unlock for access to all 40 flashcards in this deck.
Unlock Deck
k this deck
23
The form of the for loop shown below is called a(n) ____________________ for loop.
for (dataType identifier : arrayName)
statements
for (dataType identifier : arrayName)
statements
Unlock Deck
Unlock for access to all 40 flashcards in this deck.
Unlock Deck
k this deck
24
In C++, the null character is represented as ____.
A) '\0'
B) "\0"
C) '0'
D) "0"
A) '\0'
B) "\0"
C) '0'
D) "0"
Unlock Deck
Unlock for access to all 40 flashcards in this deck.
Unlock Deck
k this deck
25
The word ____________________ is used before the array declaration in a function heading to prevent the function from modifying the array.
Unlock Deck
Unlock for access to all 40 flashcards in this deck.
Unlock Deck
k this deck
26
Which of the following correctly declares and initializes alpha to be an array of four rows and three columns with the component type int?
A) int alpha[4][3] = {{0,1,2} {1,2,3} {2,3,4} {3,4,5}};
B) int alpha[4][3] = {0,1,2; 1,2,3; 2,3,4; 3,4,5};
C) int alpha[4][3] = {0,1,2: 1,2,3: 2,3,4: 3,4,5};
D) int alpha[4][3] = {{0,1,2}, {1,2,3}, {2,3,4}, {3,4,5}};
A) int alpha[4][3] = {{0,1,2} {1,2,3} {2,3,4} {3,4,5}};
B) int alpha[4][3] = {0,1,2; 1,2,3; 2,3,4; 3,4,5};
C) int alpha[4][3] = {0,1,2: 1,2,3: 2,3,4: 3,4,5};
D) int alpha[4][3] = {{0,1,2}, {1,2,3}, {2,3,4}, {3,4,5}};
Unlock Deck
Unlock for access to all 40 flashcards in this deck.
Unlock Deck
k this deck
27
Consider the following declaration: char str[15];. Which of the following statements stores "Blue Sky" into str?
A) str = "Blue Sky";
B) str[15] = "Blue Sky";
C) strcpy(str, "Blue Sky");
D) strcpy("Blue Sky");
A) str = "Blue Sky";
B) str[15] = "Blue Sky";
C) strcpy(str, "Blue Sky");
D) strcpy("Blue Sky");
Unlock Deck
Unlock for access to all 40 flashcards in this deck.
Unlock Deck
k this deck
28
Given the following declaration:
which of the following correctly finds the sum of the elements of the fifth row of sale?
A) sum = 0;
For(j = 0; j < 7; j++)
Sum = sum + sale[5][j];
B) sum = 0;
For(j = 0; j < 7; j++)
Sum = sum + sale[4][j];
C) sum = 0;
For(j = 0; j < 10; j++)
Sum = sum + sale[5][j];
D) sum = 0;
For(j = 0; j < 10; j++)
Sum = sum + sale[4][j];
![<strong>Given the following declaration: which of the following correctly finds the sum of the elements of the fifth row of sale?</strong> A) sum = 0; For(j = 0; j < 7; j++) Sum = sum + sale[5][j]; B) sum = 0; For(j = 0; j < 7; j++) Sum = sum + sale[4][j]; C) sum = 0; For(j = 0; j < 10; j++) Sum = sum + sale[5][j]; D) sum = 0; For(j = 0; j < 10; j++) Sum = sum + sale[4][j];](https://d2lvgg3v3hfg70.cloudfront.net/TB4784/11ea7810_13f6_9c29_bbd5_e1fa7a704413_TB4784_00.jpg)
A) sum = 0;
For(j = 0; j < 7; j++)
Sum = sum + sale[5][j];
B) sum = 0;
For(j = 0; j < 7; j++)
Sum = sum + sale[4][j];
C) sum = 0;
For(j = 0; j < 10; j++)
Sum = sum + sale[5][j];
D) sum = 0;
For(j = 0; j < 10; j++)
Sum = sum + sale[4][j];
Unlock Deck
Unlock for access to all 40 flashcards in this deck.
Unlock Deck
k this deck
29
Given the following declaration:
which of the following correctly finds the sum of the elements of the fourth column of sale?
A) sum = 0;
For(j = 0; j < 7; j++)
Sum = sum + sale[j][3];
B) sum = 0;
For(j = 0; j < 7; j++)
Sum = sum + sale[j][4];
C) sum = 0;
For(j = 0; j < 10; j++)
Sum = sum + sale[j][4];
D) sum = 0;
For(j = 0; j < 10; j++)
Sum = sum + sale[j][3];
![<strong>Given the following declaration: which of the following correctly finds the sum of the elements of the fourth column of sale?</strong> A) sum = 0; For(j = 0; j < 7; j++) Sum = sum + sale[j][3]; B) sum = 0; For(j = 0; j < 7; j++) Sum = sum + sale[j][4]; C) sum = 0; For(j = 0; j < 10; j++) Sum = sum + sale[j][4]; D) sum = 0; For(j = 0; j < 10; j++) Sum = sum + sale[j][3];](https://d2lvgg3v3hfg70.cloudfront.net/TB4784/11ea7810_13f6_c33a_bbd5_a90d793dfcda_TB4784_00.jpg)
A) sum = 0;
For(j = 0; j < 7; j++)
Sum = sum + sale[j][3];
B) sum = 0;
For(j = 0; j < 7; j++)
Sum = sum + sale[j][4];
C) sum = 0;
For(j = 0; j < 10; j++)
Sum = sum + sale[j][4];
D) sum = 0;
For(j = 0; j < 10; j++)
Sum = sum + sale[j][3];
Unlock Deck
Unlock for access to all 40 flashcards in this deck.
Unlock Deck
k this deck
30
Consider the following declaration: char charArray[51];
Char discard;
Assume that the input is:
Hello There!
How are you?
What is the value of discard after the following statements execute?
Cin)get(charArray, 51);
Cin)get(discard);
A) discard = ' ' (Space)
B) discard = '!'
C) discard = '\n'
D) discard = '\0'
Char discard;
Assume that the input is:
Hello There!
How are you?
What is the value of discard after the following statements execute?
Cin)get(charArray, 51);
Cin)get(discard);
A) discard = ' ' (Space)
B) discard = '!'
C) discard = '\n'
D) discard = '\0'
Unlock Deck
Unlock for access to all 40 flashcards in this deck.
Unlock Deck
k this deck
31
Consider the following declaration: int alpha[5] = {3, 5, 7, 9, 11};. Which of the following is equivalent to this statement?
A) int alpha[] = {3, 5, 7, 9, 11};
B) int alpha[] = {3 5 7 9 11};
C) int alpha[5] = [3, 5, 7, 9, 11];
D) int alpha[] = (3, 5, 7, 9, 11);
A) int alpha[] = {3, 5, 7, 9, 11};
B) int alpha[] = {3 5 7 9 11};
C) int alpha[5] = [3, 5, 7, 9, 11];
D) int alpha[] = (3, 5, 7, 9, 11);
Unlock Deck
Unlock for access to all 40 flashcards in this deck.
Unlock Deck
k this deck
32
Consider the statement int list[10][8];. Which of the following about list is true?
A) list has 10 rows and 8 columns.
B) list has 8 rows and 10 columns.
C) list has a total of 18 components.
D) list has a total of 108 components.
A) list has 10 rows and 8 columns.
B) list has 8 rows and 10 columns.
C) list has a total of 18 components.
D) list has a total of 108 components.
Unlock Deck
Unlock for access to all 40 flashcards in this deck.
Unlock Deck
k this deck
33
Consider the following statement: int alpha[25][10];. Which of the following statements about alpha is true?
A) Rows of alpha are numbered 0...24 and columns are numbered 0...9.
B) Rows of alpha are numbered 0...24 and columns are numbered 1...10.
C) Rows of alpha are numbered 1...24 and columns are numbered 0...9.
D) Rows of alpha are numbered 1...25 and columns are numbered 1...10.
A) Rows of alpha are numbered 0...24 and columns are numbered 0...9.
B) Rows of alpha are numbered 0...24 and columns are numbered 1...10.
C) Rows of alpha are numbered 1...24 and columns are numbered 0...9.
D) Rows of alpha are numbered 1...25 and columns are numbered 1...10.
Unlock Deck
Unlock for access to all 40 flashcards in this deck.
Unlock Deck
k this deck
34
Suppose that gamma is an array of 50 components of type int and j is an int variable. Which of the following for loops sets the index of gamma out of bounds?
A) for (j = 0; j <= 49; j++)
Cout << gamma[j] << " ";
B) for (j = 1; j < 50; j++)
Cout << gamma[j] << " ";
C) for (j = 0; j <= 50; j++)
Cout << gamma[j] << " ";
D) for (j = 0; j <= 48; j++)
Cout << gamma[j] << " ";
A) for (j = 0; j <= 49; j++)
Cout << gamma[j] << " ";
B) for (j = 1; j < 50; j++)
Cout << gamma[j] << " ";
C) for (j = 0; j <= 50; j++)
Cout << gamma[j] << " ";
D) for (j = 0; j <= 48; j++)
Cout << gamma[j] << " ";
Unlock Deck
Unlock for access to all 40 flashcards in this deck.
Unlock Deck
k this deck
35
Which of the following correctly declares name to be a character array and stores "William" in it?
A) char name[6] = "William";
B) char name[7] = "William";
C) char name[8] = "William";
D) char name[8] = 'William';
A) char name[6] = "William";
B) char name[7] = "William";
C) char name[8] = "William";
D) char name[8] = 'William';
Unlock Deck
Unlock for access to all 40 flashcards in this deck.
Unlock Deck
k this deck
36
A collection of a fixed number of elements (called components) arranged in n dimensions (n >= 1) is called a(n) ____.
A) matrix
B) vector
C) n-dimensional array
D) parallel array
A) matrix
B) vector
C) n-dimensional array
D) parallel array
Unlock Deck
Unlock for access to all 40 flashcards in this deck.
Unlock Deck
k this deck
37
After the following statements execute, what are the contents of matrix? 
A) 0 0
1 1
2 2
B) 0 1
2 3
4 5
C) 0 1
1 2
2 3
D) 1 1
2 2
3 3

A) 0 0
1 1
2 2
B) 0 1
2 3
4 5
C) 0 1
1 2
2 3
D) 1 1
2 2
3 3
Unlock Deck
Unlock for access to all 40 flashcards in this deck.
Unlock Deck
k this deck
38
Consider the following statement: double alpha[10][5];. The number of components of alpha is ____.
A) 15
B) 50
C) 100
D) 150
A) 15
B) 50
C) 100
D) 150
Unlock Deck
Unlock for access to all 40 flashcards in this deck.
Unlock Deck
k this deck
39
In row order form, the ____.
A) first row is stored first
B) first row is stored last
C) first column is stored first
D) first column is stored last
A) first row is stored first
B) first row is stored last
C) first column is stored first
D) first column is stored last
Unlock Deck
Unlock for access to all 40 flashcards in this deck.
Unlock Deck
k this deck
40
In a(n) ____________________ data type, each data item is a collection of other data items.
Unlock Deck
Unlock for access to all 40 flashcards in this deck.
Unlock Deck
k this deck